Note
Go to the end to download the full example code.
Buckling of beer can example#
This example is inspired by the “Buckling of Beer Can” example on the LS-DYNA Knowledge Base site. It shows how to use PyDyna to create a keyword file for LS-DYNA and then solve it from Python.
Perform required imports#
Import required packages, including those for the keywords, deck, and solver.
import os
import shutil
import subprocess
import tempfile
import numpy as np
import pandas as pd
from ansys.dyna.core import Deck
from ansys.dyna.core import keywords as kwd
from ansys.dyna.core.pre.examples.download_utilities import EXAMPLES_PATH, DownloadManager
from ansys.dyna.core.run import MemoryUnit, MpiOption, run_dyna
rundir = tempfile.TemporaryDirectory()
mesh_file_name = "mesh.k"
mesh_file = DownloadManager().download_file(
mesh_file_name, "ls-dyna", "Buckling_Beer_Can", destination=os.path.join(EXAMPLES_PATH, "Buckling_Beer_Can")
)
dynafile = "beer_can.k"
Create a deck and keywords#
Create a deck, which is the container for all the keywords. Then, create and append individual keywords to the deck.
def write_deck(filepath):
deck = Deck()
# Append control keywords
contact_auto = kwd.ContactAutomaticSingleSurfaceMortar(cid=1)
contact_auto.options["ID"].active = True
contact_auto.heading = "Single-Surface Mortar Contact (The New Explicit/Implicit Standard)"
deck.extend(
[
contact_auto,
kwd.ControlAccuracy(iacc=1),
kwd.ControlImplicitAuto(iauto=1, dtmax=0.01),
kwd.ControlImplicitDynamics(imass=1, gamma=0.6, beta=0.38),
kwd.ControlImplicitGeneral(imflag=1, dt0=0.01),
kwd.ControlImplicitSolution(nlprint=2),
kwd.ControlShell(esort=2, theory=-16, intgrd=1, nfail4=1, irquad=0),
kwd.ControlTermination(endtim=1.0),
]
)
# Append database keywords
deck.extend(
[
kwd.DatabaseGlstat(dt=1.0e-4, binary=3, ioopt=0),
kwd.DatabaseSpcforc(dt=1e-4, binary=3, ioopt=0),
kwd.DatabaseBinaryD3Plot(dt=1.0e-4),
kwd.DatabaseExtentBinary(maxint=-3, nintsld=1),
]
)
# Part keywords
can_part = kwd.Part(heading="Beer Can", pid=1, secid=1, mid=1, eosid=0)
floor_part = kwd.Part(heading="Floor", pid=2, secid=2, mid=1)
# Material keywords
mat_elastic = kwd.MatElastic(mid=1, ro=2.59e-4, e=1.0e7, pr=0.33, title="Aluminum")
mat_elastic.options["TITLE"].active = True
# Section keywords
can_shell = kwd.SectionShell(secid=1, elform=-16, shrf=0.8333, nip=3, t1=0.002, propt=0.0, title="Beer Can")
can_shell.options["TITLE"].active = True
floor_shell = kwd.SectionShell(secid=2, elform=-16, shrf=0.833, t1=0.01, propt=0.0)
floor_shell.options["TITLE"].active = True
floor_shell.title = "Floor - Just for Contact (Rigid Wall Would Have Worked Also)"
deck.extend(
[
can_part,
can_shell,
floor_part,
floor_shell,
mat_elastic,
]
)
# Load curve
load_curve = kwd.DefineCurve(lcid=1, curves=pd.DataFrame({"a1": [0.00, 1.00], "o1": [0.0, 1.000]}))
load_curve.options["TITLE"].active = True
load_curve.title = "Load vs. Time"
deck.append(load_curve)
# Define boundary conditions
load_nodes = [
50,
621,
670,
671,
672,
673,
674,
675,
676,
677,
678,
679,
680,
681,
682,
683,
684,
685,
686,
687,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
1229,
1230,
1231,
1232,
1233,
1234,
1235,
1236,
1237,
1238,
1239,
1240,
1241,
1242,
1243,
1244,
1245,
1246,
1247,
1799,
1800,
1801,
1802,
1803,
1804,
1805,
1806,
1807,
1808,
1809,
1810,
1811,
1812,
1813,
1814,
1815,
1816,
]
count = len(load_nodes)
zeros = np.zeros(count)
load_node_point = kwd.LoadNodePoint(
nodes=pd.DataFrame(
{
"nid": load_nodes,
"dof": np.full((count), 3),
"lcid": np.full((count), 1),
"sf": np.full((count), -13.1579),
"cid": zeros,
"m1": zeros,
"m2": zeros,
"m3": zeros,
}
)
)
deck.append(load_node_point)
nid = [
1,
31,
32,
33,
34,
35,
36,
37,
38,
39,
40,
41,
42,
43,
44,
45,
46,
47,
48,
49,
50,
80,
81,
82,
83,
84,
85,
86,
87,
88,
89,
90,
91,
92,
93,
94,
95,
96,
97,
98,
621,
651,
652,
653,
654,
655,
656,
657,
658,
659,
660,
661,
662,
663,
664,
665,
666,
667,
668,
669,
670,
671,
672,
673,
674,
675,
676,
677,
678,
679,
680,
681,
682,
683,
684,
685,
686,
687,
1210,
1211,
1212,
1213,
1214,
1215,
1216,
1217,
1218,
1219,
1220,
1221,
1222,
1223,
1224,
1225,
1226,
1227,
1228,
1229,
1230,
1231,
1232,
1233,
1234,
1235,
1236,
1237,
1238,
1239,
1240,
1241,
1242,
1243,
1244,
1245,
1246,
1247,
1799,
1800,
1801,
1802,
1803,
1804,
1805,
1806,
1807,
1808,
1809,
1810,
1811,
1812,
1813,
1814,
1815,
1816,
1817,
1818,
1819,
1820,
1821,
1822,
1823,
1824,
1825,
1826,
1827,
1828,
1829,
1830,
1831,
1832,
1833,
1834,
]
count = len(nid)
zeros = np.zeros(count)
ones = np.full((count), 1)
dofz = [
1,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
0,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
0,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
1,
]
boundary_spc_node = kwd.BoundarySpcNode(
nodes=pd.DataFrame(
{
"nid": nid,
"cid": zeros,
"dofx": ones,
"dofy": ones,
"dofz": dofz,
"dofrx": ones,
"dofry": ones,
"dofrz": ones,
}
)
)
deck.append(boundary_spc_node)
# Define nodes and elements
deck.append(kwd.Include(filename=mesh_file_name))
deck.export_file(filepath)
return deck
def run_post(filepath):
pass
shutil.copy(mesh_file, os.path.join(rundir.name, mesh_file_name))
deck = write_deck(os.path.join(rundir.name, dynafile))
View the model#
You can use the PyVista plot
method in the deck
class to view
the model.
deck.plot(cwd=rundir.name)
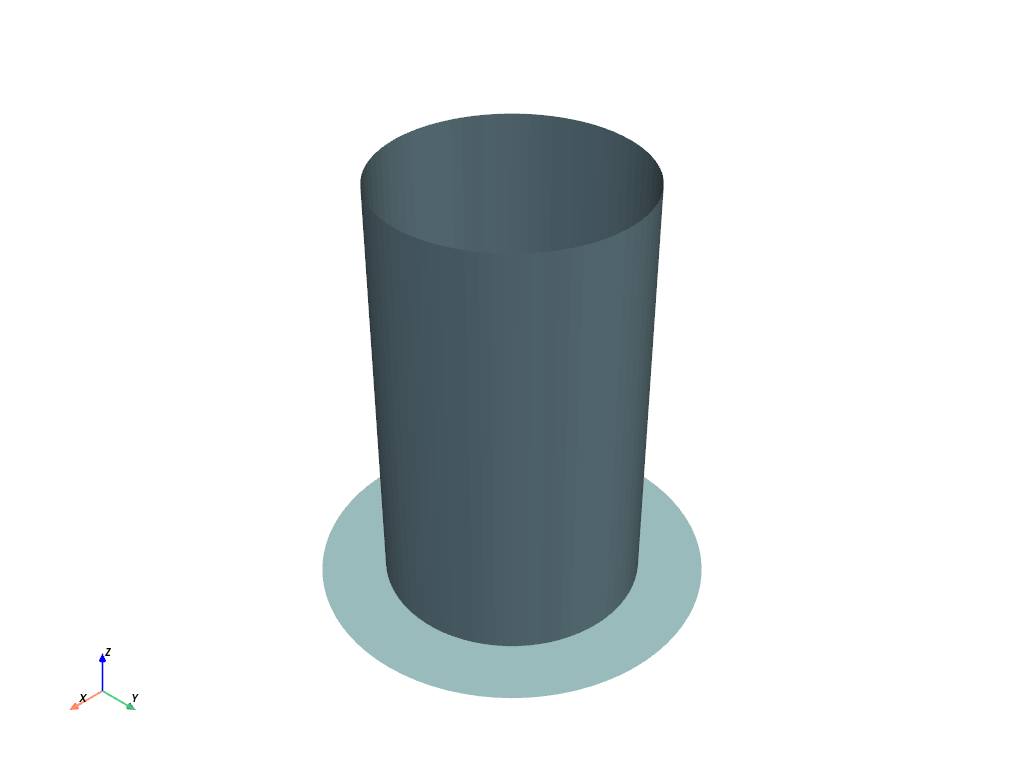
Run the Dyna solver#
try:
run_dyna(
dynafile,
working_directory=rundir.name,
ncpu=2,
mpi_option=MpiOption.MPP_INTEL_MPI,
memory=20,
memory_unit=MemoryUnit.MB,
)
except subprocess.CalledProcessError:
# this example doesn't run to completion because it is a highly nonlinear buckling
pass
run_post(rundir.name)
License option : check ansys licenses only
***************************************************************
* ANSYS LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2023 ANSYS, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of ANSYS, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the ANSYS, Inc. online documentation or the ANSYS, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* ANSYS, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* ANSYS, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the ANSYS, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the ANSYS, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
Date: 06/26/2025 Time: 18:12:40
___________________________________________________
| |
| LS-DYNA, A Program for Nonlinear Dynamic |
| Analysis of Structures in Three Dimensions |
| Date : 10/16/2023 Time: 19:29:09 |
| Version : mpp d R14 |
| Revision: R14.1-205-geb5348f751 |
| AnLicVer: 2024 R1 (20231025+2752148) |
| |
| Features enabled in this version: |
| Distributed Memory Parallel |
| CESE CHEMISTRY EM ICFD STOCHASTIC_PARTICLES |
| FFTW (multi-dimensional FFTW Library) |
| ANSYSLIC enabled |
| |
| Platform : Intel-MPI 2018 Xeon64 |
| OS Level : Linux CentOS 7.9 uum |
| Compiler : Intel Fortran Compiler 19.0 SSE2 |
| Hostname : ebf4d118d8e6 |
| Precision : Double precision (I8R8) |
| |
| Unauthorized use infringes Ansys Inc. copyrights |
|___________________________________________________|
[license/info] Successfully checked out 2 of "dyna_solver_core".
[license/info] --> Checkout ID: ebf4d118d8e6-root-23-000004 (days left: 292)
[license/info] --> Customer ID: 0
[license/info] Successfully started "LSDYNA (Core-based License)".
Executing with ANSYS license
Command line options: i=beer_can.k
memory=20m
Input file: beer_can.k
The native file format : 64-bit small endian
Memory size from command line: 20000000, 0
Memory size from command line: 20000000, 20000000
Memory for the head node
Memory installed (MB) : 32098
Memory available (MB) : 29987
on UNIX computers note the following change:
ctrl-c interrupts ls-dyna and prompts for a sense switch.
type the desired sense switch: sw1., sw2., etc. to continue
the execution. ls-dyna will respond as explained in the users manual
type response
----- ------------------------------------------------------------
quit ls-dyna terminates.
stop ls-dyna terminates.
sw1. a restart file is written and ls-dyna terminates.
sw2. ls-dyna responds with time and cycle numbers.
sw3. a restart file is written and ls-dyna continues calculations.
sw4. a plot state is written and ls-dyna continues calculations.
sw5. ls-dyna enters interactive graphics phase.
swa. ls-dyna flushes all output i/o buffers.
swb. a dynain is written and ls-dyna continues calculations.
swc. a restart and dynain are written and ls-dyna continues calculations.
swd. a restart and dynain are written and ls-dyna terminates.
swe. stop dynamic relaxation just as though convergence
endtime=time change the termination time
lpri toggle implicit lin. alg. solver output on/off.
nlpr toggle implicit nonlinear solver output on/off.
iter toggle implicit output to d3iter database on/off.
prof output timing data to prof.out and continue.
conv force implicit nonlinear convergence for current time step.
ttrm terminate implicit time step, reduce time step, retry time step.
rtrm terminate implicit at end of current time step.
******** notice ******** notice ******** notice ********
* *
* This is the LS-DYNA Finite Element code. *
* *
* Neither LST nor the authors assume any responsibility for *
* the validity, accuracy, or applicability of any results *
* obtained from this system. Users must verify their own *
* results. *
* *
* LST endeavors to make the LS-DYNA code as complete, *
* accurate and easy to use as possible. *
* Suggestions and comments are welcomed. Please report any *
* errors encountered in either the documentation or results *
* immediately to LST through your site focus. *
* *
* Copyright (C) 1990-2021 *
* by Livermore Software Technology, LLC *
* All rights reserved *
* *
******** notice ******** notice ******** notice ********
Beginning of keyword reader 06/26/25 18:12:47
06/26/25 18:12:47
Open include file: mesh.k
Memory required to process keyword : 285092
Additional dynamic memory required : 2394491
MPP execution with 2 procs
Initial reading of file 06/26/25 18:12:47
Implicit dynamics is now active
Performing Decomposition -- Phase 1 06/26/25 18:12:47
Performing Recursive Coordinate Bisection (RCB)
Memory required for decomposition : 53592
Additional dynamic memory required : 2559869
Performing Decomposition -- Phase 2 06/26/25 18:12:47
Performing Decomposition -- Phase 3 06/26/25 18:12:47
Implicit dynamics is now active
input of data is completed
initial kinetic energy = 0.00000000E+00
The LS-DYNA time step size should not exceed 1.656E-07
to avoid contact instabilities. If the step size is
bigger then scale the penalty of the offending surface.
Implicit dynamics is now active
termination time = 1.000E+00
The following binary output files are being created,
and contain data equivalent to the indicated ascii output files
binout0000: (on processor 0)
glstat
spcforc
Memory required to begin solution (memory= 320K)
Minimum 281K on processor 1
Maximum 320K on processor 0
Average 301K
Matrix Assembly dynamically allocated memory
Maximum 160K
Additional dynamically allocated memory
Minimum 4056K on processor 1
Maximum 4374K on processor 0
Average 4215K
Total allocated memory
Minimum 4496K on processor 1
Maximum 4854K on processor 0
Average 4675K
initialization completed
calculation with mass scaling for minimum dt
added mass = 0.0000E+00
physical mass= 6.5553E-05
ratio = 0.0000E+00
1 t 0.0000E+00 dt 1.00E-02 flush i/o buffers 06/26/25 18:12:47
1 t 0.0000E+00 dt 1.00E-02 write d3plot file 06/26/25 18:12:47
Implicit dynamics is now active
BEGIN implicit dynamics step 1 t= 1.0000E-02 06/26/25 18:12:47
============================================================
time = 1.00000E-02
current step size = 1.00000E-02
================================================
== IMPLICIT USAGE ALERT ==
================================================
== Memory Management for Implicit has changed ==
== after R10. Please use: ==
== memory= 1M memory2= 1M ==
================================================
Iteration: 1 *|du|/|u| = 1.0000000E+00 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.2021998E-04 *Ei/E0 = 2.0305868E-07
Equilibrium established after 2 iterations 06/26/25 18:12:47
estimated total cpu time = 38 sec ( 0 hrs 0 mins)
estimated cpu time to complete = 38 sec ( 0 hrs 0 mins)
estimated total clock time = 45 sec ( 0 hrs 0 mins)
estimated clock time to complete = 38 sec ( 0 hrs 0 mins)
6 t 1.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:47
BEGIN implicit dynamics step 2 t= 2.0000E-02 06/26/25 18:12:47
============================================================
time = 2.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 5.0000250E-01 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.2522434E-04 *Ei/E0 = 9.9652787E-07
Equilibrium established after 2 iterations 06/26/25 18:12:48
10 t 2.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:48
BEGIN implicit dynamics step 3 t= 3.0000E-02 06/26/25 18:12:48
============================================================
time = 3.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.3332563E-01 *Ei/E0 = 9.9996901E-01
Iteration: 2 *|du|/|u| = 3.3075191E-04 *Ei/E0 = 2.4420705E-06
Equilibrium established after 2 iterations 06/26/25 18:12:48
14 t 3.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:48
BEGIN implicit dynamics step 4 t= 4.0000E-02 06/26/25 18:12:48
============================================================
time = 4.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 2.4999550E-01 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.3675018E-04 *Ei/E0 = 4.6079789E-06
Equilibrium established after 2 iterations 06/26/25 18:12:48
18 t 4.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:48
BEGIN implicit dynamics step 5 t= 5.0000E-02 06/26/25 18:12:48
============================================================
time = 5.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 1.9999607E-01 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.4322009E-04 *Ei/E0 = 7.5743634E-06
Equilibrium established after 2 iterations 06/26/25 18:12:48
22 t 5.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:48
BEGIN implicit dynamics step 6 t= 6.0000E-02 06/26/25 18:12:48
============================================================
time = 6.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 1.6665977E-01 *Ei/E0 = 9.9999171E-01
Iteration: 2 *|du|/|u| = 3.5019120E-04 *Ei/E0 = 1.1436154E-05
Equilibrium established after 2 iterations 06/26/25 18:12:49
26 t 6.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:49
BEGIN implicit dynamics step 7 t= 7.0000E-02 06/26/25 18:12:49
============================================================
time = 7.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 1.4285164E-01 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.5773447E-04 *Ei/E0 = 1.6307565E-05
Equilibrium established after 2 iterations 06/26/25 18:12:49
30 t 7.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:49
BEGIN implicit dynamics step 8 t= 8.0000E-02 06/26/25 18:12:49
============================================================
time = 8.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 1.2499492E-01 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.6588923E-04 *Ei/E0 = 2.2323129E-05
Equilibrium established after 2 iterations 06/26/25 18:12:49
34 t 8.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:49
BEGIN implicit dynamics step 9 t= 9.0000E-02 06/26/25 18:12:49
============================================================
time = 9.00000E-02
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 1.1110503E-01 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.7471370E-04 *Ei/E0 = 2.9644269E-05
Equilibrium established after 2 iterations 06/26/25 18:12:49
estimated total cpu time = 25 sec ( 0 hrs 0 mins)
estimated cpu time to complete = 23 sec ( 0 hrs 0 mins)
estimated total clock time = 32 sec ( 0 hrs 0 mins)
estimated clock time to complete = 23 sec ( 0 hrs 0 mins)
38 t 9.0000E-02 dt 1.00E-02 write d3plot file 06/26/25 18:12:49
BEGIN implicit dynamics step 10 t= 1.0000E-01 06/26/25 18:12:49
============================================================
time = 1.00000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 9.9994793E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.8429631E-04 *Ei/E0 = 3.8466486E-05
Equilibrium established after 2 iterations 06/26/25 18:12:50
42 t 1.0000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:50
BEGIN implicit dynamics step 11 t= 1.1000E-01 06/26/25 18:12:50
============================================================
time = 1.10000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 9.0904278E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 3.9471113E-04 *Ei/E0 = 4.9024578E-05
Equilibrium established after 2 iterations 06/26/25 18:12:50
46 t 1.1000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:50
BEGIN implicit dynamics step 12 t= 1.2000E-01 06/26/25 18:12:50
============================================================
time = 1.20000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 8.3328303E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.0605414E-04 *Ei/E0 = 6.1604220E-05
Equilibrium established after 2 iterations 06/26/25 18:12:50
50 t 1.2000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:50
BEGIN implicit dynamics step 13 t= 1.3000E-01 06/26/25 18:12:50
============================================================
time = 1.30000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 7.6918751E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.1845031E-04 *Ei/E0 = 7.6556889E-05
Equilibrium established after 2 iterations 06/26/25 18:12:50
54 t 1.3000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:50
BEGIN implicit dynamics step 14 t= 1.4000E-01 06/26/25 18:12:50
============================================================
time = 1.40000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 7.1424697E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.3202769E-04 *Ei/E0 = 9.4313877E-05
Equilibrium established after 2 iterations 06/26/25 18:12:51
58 t 1.4000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:51
BEGIN implicit dynamics step 15 t= 1.5000E-01 06/26/25 18:12:51
============================================================
time = 1.50000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 6.6662985E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.4694473E-04 *Ei/E0 = 1.1541148E-04
Equilibrium established after 2 iterations 06/26/25 18:12:51
62 t 1.5000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:51
BEGIN implicit dynamics step 16 t= 1.6000E-01 06/26/25 18:12:51
============================================================
time = 1.60000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 6.2497003E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.6339728E-04 *Ei/E0 = 1.4052308E-04
Equilibrium established after 2 iterations 06/26/25 18:12:51
66 t 1.6000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:51
BEGIN implicit dynamics step 17 t= 1.7000E-01 06/26/25 18:12:51
============================================================
time = 1.70000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 5.8821090E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.8160702E-04 *Ei/E0 = 1.7049797E-04
Equilibrium established after 2 iterations 06/26/25 18:12:51
70 t 1.7000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:51
BEGIN implicit dynamics step 18 t= 1.8000E-01 06/26/25 18:12:51
============================================================
time = 1.80000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 5.5553579E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 5.0184571E-04 *Ei/E0 = 2.0642066E-04
Equilibrium established after 2 iterations 06/26/25 18:12:52
74 t 1.8000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:52
BEGIN implicit dynamics step 19 t= 1.9000E-01 06/26/25 18:12:52
============================================================
time = 1.90000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 5.2630351E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 5.2444697E-04 *Ei/E0 = 2.4969127E-04
Equilibrium established after 2 iterations 06/26/25 18:12:52
78 t 1.9000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:52
BEGIN implicit dynamics step 20 t= 2.0000E-01 06/26/25 18:12:52
============================================================
time = 2.00000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 4.9999469E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 5.4980972E-04 *Ei/E0 = 3.0213427E-04
Equilibrium established after 2 iterations 06/26/25 18:12:52
82 t 2.0000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:52
BEGIN implicit dynamics step 21 t= 2.1000E-01 06/26/25 18:12:52
============================================================
time = 2.10000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 4.7619198E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 5.7842801E-04 *Ei/E0 = 3.6615842E-04
Equilibrium established after 2 iterations 06/26/25 18:12:52
86 t 2.1000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:52
BEGIN implicit dynamics step 22 t= 2.2000E-01 06/26/25 18:12:52
============================================================
time = 2.20000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 4.5455558E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 6.1091730E-04 *Ei/E0 = 4.4498580E-04
Equilibrium established after 2 iterations 06/26/25 18:12:53
90 t 2.2000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:53
BEGIN implicit dynamics step 23 t= 2.3000E-01 06/26/25 18:12:53
============================================================
time = 2.30000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 4.3480125E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 6.4804294E-04 *Ei/E0 = 5.4298441E-04
Equilibrium established after 2 iterations 06/26/25 18:12:53
94 t 2.3000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:53
BEGIN implicit dynamics step 24 t= 2.4000E-01 06/26/25 18:12:53
============================================================
time = 2.40000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 4.1669394E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 6.9077669E-04 *Ei/E0 = 6.6616644E-04
Equilibrium established after 2 iterations 06/26/25 18:12:53
98 t 2.4000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:53
BEGIN implicit dynamics step 25 t= 2.5000E-01 06/26/25 18:12:53
============================================================
time = 2.50000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 4.0003696E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 7.4036921E-04 *Ei/E0 = 8.2293943E-04
Equilibrium established after 2 iterations 06/26/25 18:12:53
102 t 2.5000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:53
BEGIN implicit dynamics step 26 t= 2.6000E-01 06/26/25 18:12:53
============================================================
time = 2.60000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.8466177E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 7.9846254E-04 *Ei/E0 = 1.0252701E-03
Equilibrium established after 2 iterations 06/26/25 18:12:54
106 t 2.6000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:54
BEGIN implicit dynamics step 27 t= 2.7000E-01 06/26/25 18:12:54
============================================================
time = 2.70000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.7042582E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 8.6732233E-04 *Ei/E0 = 1.2905077E-03
Equilibrium established after 2 iterations 06/26/25 18:12:54
110 t 2.7000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:54
BEGIN implicit dynamics step 28 t= 2.8000E-01 06/26/25 18:12:54
============================================================
time = 2.80000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.5720698E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 9.5043245E-04 *Ei/E0 = 1.6443215E-03
Equilibrium established after 2 iterations 06/26/25 18:12:54
114 t 2.8000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:54
BEGIN implicit dynamics step 29 t= 2.9000E-01 06/26/25 18:12:54
============================================================
time = 2.90000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.4489877E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 1.0546097E-03 *Ei/E0 = 2.1255588E-03
Equilibrium established after 2 iterations 06/26/25 18:12:54
118 t 2.9000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:54
BEGIN implicit dynamics step 30 t= 3.0000E-01 06/26/25 18:12:54
============================================================
time = 3.00000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.3341080E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 1.1993864E-03 *Ei/E0 = 2.7945527E-03
Equilibrium established after 2 iterations 06/26/25 18:12:55
122 t 3.0000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:55
BEGIN implicit dynamics step 31 t= 3.1000E-01 06/26/25 18:12:55
============================================================
time = 3.10000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.2267524E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 1.4625222E-03 *Ei/E0 = 3.7483755E-03
Equilibrium established after 2 iterations 06/26/25 18:12:55
126 t 3.1000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:55
BEGIN implicit dynamics step 32 t= 3.2000E-01 06/26/25 18:12:55
============================================================
time = 3.20000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.1270930E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 2.1772529E-03 *Ei/E0 = 5.1543716E-03
Equilibrium established after 2 iterations 06/26/25 18:12:55
130 t 3.2000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:55
BEGIN implicit dynamics step 33 t= 3.3000E-01 06/26/25 18:12:55
============================================================
time = 3.30000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.0410595E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 4.5139313E-03 *Ei/E0 = 7.3650789E-03
Equilibrium established after 2 iterations 06/26/25 18:12:55
134 t 3.3000E-01 dt 1.00E-02 write d3plot file 06/26/25 18:12:55
BEGIN implicit dynamics step 34 t= 3.4000E-01 06/26/25 18:12:55
============================================================
time = 3.40000E-01
current step size = 1.00000E-02
Iteration: 1 *|du|/|u| = 3.0212011E-02 *Ei/E0 = 1.0000000E+00
Iteration: 2 *|du|/|u| = 1.1995946E-02 *Ei/E0 = 1.1650989E-02
Iteration: 3 *|du|/|u| = 2.1795328E-01 *Ei/E0 = 1.3711541E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.519E+01 exceeds prior maximum 1.134E+01
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 5.9801859E-02 *Ei/E0 = 4.0841643E-02
Iteration: 5 *|du|/|u| = 8.7798331E-02 *Ei/E0 = 6.9588163E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.071E+01 exceeds prior maximum 1.519E+01
automatically REFORMING stiffness matrix...
Iteration: 6 *|du|/|u| = 1.6184543E-01 *Ei/E0 = 3.2401520E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.179E+01 exceeds prior maximum 5.071E+01
automatically REFORMING stiffness matrix...
Iteration: 7 *|du|/|u| = 2.7859446E-01 *Ei/E0 = 1.0209179E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.698E+02 exceeds prior maximum 8.179E+01
automatically REFORMING stiffness matrix...
Iteration: 8 *|du|/|u| = 4.6561450E-01 *Ei/E0 = 3.8854895E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.685E+02 exceeds prior maximum 1.698E+02
automatically REFORMING stiffness matrix...
Iteration: 9 *|du|/|u| = 7.5555714E-01 *Ei/E0 = 1.1795494E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.471E+02 exceeds prior maximum 2.685E+02
automatically REFORMING stiffness matrix...
Iteration: 10 *|du|/|u| = 1.0664892E+00 *Ei/E0 = 2.8372458E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.081E+02 exceeds prior maximum 3.471E+02
automatically REFORMING stiffness matrix...
Iteration: 11 *|du|/|u| = 1.4427977E+00 *Ei/E0 = 7.3394052E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.273E+02 exceeds prior maximum 5.081E+02
automatically REFORMING stiffness matrix...
Iteration: 12 *|du|/|u| = 1.6615012E+00 *Ei/E0 = 1.4500346E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.471E+02 exceeds prior maximum 7.273E+02
automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.8194866E+00 *Ei/E0 = 2.3447779E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.147E+03 exceeds prior maximum 9.471E+02
automatically REFORMING stiffness matrix...
Iteration: 14 *|du|/|u| = 1.9371527E+00 *Ei/E0 = 3.4817929E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.327E+03 exceeds prior maximum 1.147E+03
automatically REFORMING stiffness matrix...
Iteration: 15 *|du|/|u| = 1.8224002E+00 *Ei/E0 = 4.7837642E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.528E+03 exceeds prior maximum 1.327E+03
automatically REFORMING stiffness matrix...
Iteration: 16 *|du|/|u| = 1.6131625E+00 *Ei/E0 = 6.0426251E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.781E+03 exceeds prior maximum 1.528E+03
automatically REFORMING stiffness matrix...
Iteration: 17 *|du|/|u| = 1.4999749E+00 *Ei/E0 = 7.5755546E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.070E+03 exceeds prior maximum 1.781E+03
automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 1.1519778E+00 *Ei/E0 = 8.8187593E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.458E+03 exceeds prior maximum 2.070E+03
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 9.3084674E-01 *Ei/E0 = 9.6865025E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.907E+03 exceeds prior maximum 2.458E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.00000E-02 dt(new) = 4.64159E-03
------------------------------------------------------------
BEGIN implicit dynamics step 34 t= 3.3464E-01 06/26/25 18:13:00
============================================================
time = 3.34642E-01
current step size = 4.64159E-03
Iteration: 1 *|du|/|u| = 1.5449475E-02 *Ei/E0 = 2.1767331E-01
Iteration: 2 *|du|/|u| = 1.1763330E-02 *Ei/E0 = 7.1275424E-03
Iteration: 3 *|du|/|u| = 2.9281596E-01 *Ei/E0 = 1.2834658E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.135E+01 exceeds prior maximum 5.978E+00
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 5.0443240E-02 *Ei/E0 = 2.6425124E-02
Iteration: 5 *|du|/|u| = 7.5428612E-02 *Ei/E0 = 4.7167334E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.553E+01 exceeds prior maximum 1.135E+01
automatically REFORMING stiffness matrix...
Iteration: 6 *|du|/|u| = 1.3557902E-01 *Ei/E0 = 1.8778579E-01
Iteration: 7 *|du|/|u| = 1.3244810E-01 *Ei/E0 = 1.9367676E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.600E+01 exceeds prior maximum 3.553E+01
automatically REFORMING stiffness matrix...
Iteration: 8 *|du|/|u| = 2.5226957E-01 *Ei/E0 = 9.0283587E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.596E+02 exceeds prior maximum 8.600E+01
automatically REFORMING stiffness matrix...
Iteration: 9 *|du|/|u| = 4.2294789E-01 *Ei/E0 = 3.1042933E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.521E+02 exceeds prior maximum 1.596E+02
automatically REFORMING stiffness matrix...
Iteration: 10 *|du|/|u| = 6.8521792E-01 *Ei/E0 = 9.5150646E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.378E+02 exceeds prior maximum 2.521E+02
automatically REFORMING stiffness matrix...
Iteration: 11 *|du|/|u| = 9.9970674E-01 *Ei/E0 = 2.4379758E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.922E+02 exceeds prior maximum 3.378E+02
automatically REFORMING stiffness matrix...
Iteration: 12 *|du|/|u| = 1.4117000E+00 *Ei/E0 = 6.4898978E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.422E+02 exceeds prior maximum 4.922E+02
automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.5966933E+00 *Ei/E0 = 1.1993087E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.698E+02 exceeds prior maximum 6.422E+02
automatically REFORMING stiffness matrix...
Iteration: 14 *|du|/|u| = 1.7638907E+00 *Ei/E0 = 2.0379205E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.077E+03 exceeds prior maximum 8.698E+02
automatically REFORMING stiffness matrix...
Iteration: 15 *|du|/|u| = 1.9017712E+00 *Ei/E0 = 3.1019437E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.260E+03 exceeds prior maximum 1.077E+03
automatically REFORMING stiffness matrix...
Iteration: 16 *|du|/|u| = 1.8438792E+00 *Ei/E0 = 4.3795465E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.452E+03 exceeds prior maximum 1.260E+03
automatically REFORMING stiffness matrix...
Iteration: 17 *|du|/|u| = 1.6237909E+00 *Ei/E0 = 5.5926328E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.694E+03 exceeds prior maximum 1.452E+03
automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 1.5171562E+00 *Ei/E0 = 7.0572189E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.970E+03 exceeds prior maximum 1.694E+03
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 1.1869066E+00 *Ei/E0 = 8.3350344E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.330E+03 exceeds prior maximum 1.970E+03
automatically REFORMING stiffness matrix...
Iteration: 20 *|du|/|u| = 9.4678516E-01 *Ei/E0 = 9.2407792E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.744E+03 exceeds prior maximum 2.330E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 4.64159E-03 dt(new) = 2.15443E-03
------------------------------------------------------------
BEGIN implicit dynamics step 34 t= 3.3215E-01 06/26/25 18:13:04
============================================================
time = 3.32154E-01
current step size = 2.15443E-03
Iteration: 1 *|du|/|u| = 9.3017629E-03 *Ei/E0 = 4.9927129E-02
Iteration: 2 *|du|/|u| = 1.2561494E-02 *Ei/E0 = 6.1878640E-03
Iteration: 3 *|du|/|u| = 2.0349818E-01 *Ei/E0 = 7.2124065E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.211E+01 exceeds prior maximum 3.490E+00
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 4.8843026E-02 *Ei/E0 = 2.7406330E-02
Iteration: 5 *|du|/|u| = 7.1735741E-02 *Ei/E0 = 4.3159745E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.201E+01 exceeds prior maximum 1.211E+01
automatically REFORMING stiffness matrix...
Iteration: 6 *|du|/|u| = 1.2521320E-01 *Ei/E0 = 1.6080086E-01
Iteration: 7 *|du|/|u| = 1.2444184E-01 *Ei/E0 = 1.6926898E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.405E+01 exceeds prior maximum 3.201E+01
automatically REFORMING stiffness matrix...
Iteration: 8 *|du|/|u| = 2.2340983E-01 *Ei/E0 = 7.1910777E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.793E+02 exceeds prior maximum 7.405E+01
automatically REFORMING stiffness matrix...
Iteration: 9 *|du|/|u| = 4.0921107E-01 *Ei/E0 = 3.3525554E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.635E+02 exceeds prior maximum 1.793E+02
automatically REFORMING stiffness matrix...
Iteration: 10 *|du|/|u| = 6.7096754E-01 *Ei/E0 = 9.8694342E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.542E+02 exceeds prior maximum 2.635E+02
automatically REFORMING stiffness matrix...
Iteration: 11 *|du|/|u| = 9.9588914E-01 *Ei/E0 = 2.6378309E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.156E+02 exceeds prior maximum 3.542E+02
automatically REFORMING stiffness matrix...
Iteration: 12 *|du|/|u| = 1.3769236E+00 *Ei/E0 = 6.9817297E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.761E+02 exceeds prior maximum 5.156E+02
automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.5328865E+00 *Ei/E0 = 1.2666064E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.091E+02 exceeds prior maximum 6.761E+02
automatically REFORMING stiffness matrix...
Iteration: 14 *|du|/|u| = 1.6857418E+00 *Ei/E0 = 2.1197650E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.117E+03 exceeds prior maximum 9.091E+02
automatically REFORMING stiffness matrix...
Iteration: 15 *|du|/|u| = 1.8145641E+00 *Ei/E0 = 3.2028875E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.300E+03 exceeds prior maximum 1.117E+03
automatically REFORMING stiffness matrix...
Iteration: 16 *|du|/|u| = 1.7602071E+00 *Ei/E0 = 4.4951986E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.500E+03 exceeds prior maximum 1.300E+03
automatically REFORMING stiffness matrix...
Iteration: 17 *|du|/|u| = 1.5786436E+00 *Ei/E0 = 5.7603263E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.749E+03 exceeds prior maximum 1.500E+03
automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 1.4465827E+00 *Ei/E0 = 7.2597922E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.035E+03 exceeds prior maximum 1.749E+03
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 1.1043457E+00 *Ei/E0 = 8.4166085E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.417E+03 exceeds prior maximum 2.035E+03
automatically REFORMING stiffness matrix...
Iteration: 20 *|du|/|u| = 9.0996194E-01 *Ei/E0 = 9.2504944E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.858E+03 exceeds prior maximum 2.417E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 2.15443E-03 dt(new) = 1.00000E-03
------------------------------------------------------------
BEGIN implicit dynamics step 34 t= 3.3100E-01 06/26/25 18:13:09
============================================================
time = 3.31000E-01
current step size = 1.00000E-03
Iteration: 1 *|du|/|u| = 6.8355386E-03 *Ei/E0 = 1.4149769E-02
Iteration: 2 *|du|/|u| = 1.5643708E-02 *Ei/E0 = 8.1126758E-03
Iteration: 3 *|du|/|u| = 7.9631607E-02 *Ei/E0 = 2.5110866E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.013E+01 exceeds prior maximum 2.336E+00
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 3.7174424E-02 *Ei/E0 = 2.1275867E-02
Iteration: 5 *|du|/|u| = 6.0714687E+01 *Ei/E0 = 3.2964236E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.759E+01 exceeds prior maximum 1.013E+01
automatically REFORMING stiffness matrix...
Iteration: 6 *|du|/|u| = 1.1357326E-01 *Ei/E0 = 2.0969387E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.785E+01 exceeds prior maximum 3.759E+01
automatically REFORMING stiffness matrix...
Iteration: 7 *|du|/|u| = 1.7374926E-01 *Ei/E0 = 4.1650831E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.027E+02 exceeds prior maximum 3.785E+01
automatically REFORMING stiffness matrix...
Iteration: 8 *|du|/|u| = 2.8806340E-01 *Ei/E0 = 1.7230401E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.201E+02 exceeds prior maximum 1.027E+02
automatically REFORMING stiffness matrix...
Iteration: 9 *|du|/|u| = 5.2141237E-01 *Ei/E0 = 7.7258773E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.673E+02 exceeds prior maximum 2.201E+02
automatically REFORMING stiffness matrix...
Iteration: 10 *|du|/|u| = 8.8574757E-01 *Ei/E0 = 3.0214345E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.830E+02 exceeds prior maximum 3.673E+02
automatically REFORMING stiffness matrix...
Iteration: 11 *|du|/|u| = 1.1513658E+00 *Ei/E0 = 8.3374379E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.073E+02 exceeds prior maximum 5.830E+02
automatically REFORMING stiffness matrix...
Iteration: 12 *|du|/|u| = 1.2931582E+00 *Ei/E0 = 1.5229570E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.005E+03 exceeds prior maximum 8.073E+02
automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.3823944E+00 *Ei/E0 = 2.3348258E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.230E+03 exceeds prior maximum 1.005E+03
automatically REFORMING stiffness matrix...
Iteration: 14 *|du|/|u| = 1.4413715E+00 *Ei/E0 = 3.4022631E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.428E+03 exceeds prior maximum 1.230E+03
automatically REFORMING stiffness matrix...
Iteration: 15 *|du|/|u| = 1.4852155E+00 *Ei/E0 = 4.5827434E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.637E+03 exceeds prior maximum 1.428E+03
automatically REFORMING stiffness matrix...
Iteration: 16 *|du|/|u| = 1.5033367E+00 *Ei/E0 = 6.0682620E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.879E+03 exceeds prior maximum 1.637E+03
automatically REFORMING stiffness matrix...
Iteration: 17 *|du|/|u| = 1.3101451E+00 *Ei/E0 = 7.6123783E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.200E+03 exceeds prior maximum 1.879E+03
automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 1.0355990E+00 *Ei/E0 = 8.6552863E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.595E+03 exceeds prior maximum 2.200E+03
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 9.4052772E-01 *Ei/E0 = 9.4263947E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.082E+03 exceeds prior maximum 2.595E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.00000E-03 dt(new) = 4.64159E-04
------------------------------------------------------------
BEGIN implicit dynamics step 34 t= 3.3046E-01 06/26/25 18:13:14
============================================================
time = 3.30464E-01
current step size = 4.64159E-04
Iteration: 1 *|du|/|u| = 4.7558296E-03 *Ei/E0 = 6.5415126E-03
Iteration: 2 *|du|/|u| = 1.7804316E-02 *Ei/E0 = 1.3729398E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.821E+00 exceeds prior maximum 1.800E+00
automatically REFORMING stiffness matrix...
Iteration: 3 *|du|/|u| = 7.3001438E-03 *Ei/E0 = 3.2418573E-03
Iteration: 4 *|du|/|u| = 3.4349153E-02 *Ei/E0 = 8.4466235E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.190E+00 exceeds prior maximum 1.821E+00
automatically REFORMING stiffness matrix...
Iteration: 5 *|du|/|u| = 1.8093068E-02 *Ei/E0 = 1.3376128E-02
Iteration: 6 *|du|/|u| = 3.2076802E-01 *Ei/E0 = 1.9526640E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.450E+01 exceeds prior maximum 4.190E+00
automatically REFORMING stiffness matrix...
Iteration: 7 *|du|/|u| = 4.2177072E-02 *Ei/E0 = 7.8430131E-02
Iteration: 8 *|du|/|u| = 5.6371925E-02 *Ei/E0 = 1.1252521E-01
Iteration: 9 *|du|/|u| = 5.7055295E-02 *Ei/E0 = 1.1686853E-01
Iteration: 10 *|du|/|u| = 5.7712303E-02 *Ei/E0 = 1.2132394E-01
Iteration: 11 *|du|/|u| = 5.8339977E-02 *Ei/E0 = 1.2588754E-01
Iteration: 12 *|du|/|u| = 5.8935479E-02 *Ei/E0 = 1.3055513E-01
Iteration: 13 *|du|/|u| = 5.9496148E-02 *Ei/E0 = 1.3532223E-01
Iteration: 14 *|du|/|u| = 6.0019548E-02 *Ei/E0 = 1.4018423E-01
Iteration: 15 *|du|/|u| = 6.0503519E-02 *Ei/E0 = 1.4513643E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.551E+01 exceeds prior maximum 1.450E+01
automatically REFORMING stiffness matrix...
Iteration: 16 *|du|/|u| = 7.2356412E-02 *Ei/E0 = 1.8764785E-01
Iteration: 17 *|du|/|u| = 8.2483044E-02 *Ei/E0 = 2.5901837E-01
Iteration: 18 *|du|/|u| = 8.4713050E-02 *Ei/E0 = 2.7834031E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.563E+01 exceeds prior maximum 1.551E+01
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 9.4486115E-02 *Ei/E0 = 3.3646623E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.653E+01 exceeds prior maximum 1.563E+01
automatically REFORMING stiffness matrix...
Iteration: 20 *|du|/|u| = 1.1882536E-01 *Ei/E0 = 5.4945556E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.743E+01 exceeds prior maximum 1.653E+01
automatically REFORMING stiffness matrix...
Iteration: 21 *|du|/|u| = 1.4359199E-01 *Ei/E0 = 8.1807499E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.801E+01 exceeds prior maximum 1.743E+01
automatically REFORMING stiffness matrix...
Iteration: 22 *|du|/|u| = 1.5859310E-01 *Ei/E0 = 1.1208413E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.723E+02 exceeds prior maximum 1.801E+01
automatically REFORMING stiffness matrix...
Iteration: 23 *|du|/|u| = 3.9794918E-01 *Ei/E0 = 9.9813370E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.443E+02 exceeds prior maximum 1.723E+02
automatically REFORMING stiffness matrix...
Iteration: 24 *|du|/|u| = 7.4405396E-01 *Ei/E0 = 5.5659881E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.321E+02 exceeds prior maximum 4.443E+02
automatically REFORMING stiffness matrix...
Iteration: 25 *|du|/|u| = 8.3051958E-01 *Ei/E0 = 1.0583623E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.302E+02 exceeds prior maximum 6.321E+02
automatically REFORMING stiffness matrix...
Iteration: 26 *|du|/|u| = 8.6156900E-01 *Ei/E0 = 1.5723207E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.143E+03 exceeds prior maximum 8.302E+02
automatically REFORMING stiffness matrix...
Iteration: 27 *|du|/|u| = 9.1982911E-01 *Ei/E0 = 2.2542115E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.687E+03 exceeds prior maximum 1.143E+03
automatically REFORMING stiffness matrix...
Iteration: 28 *|du|/|u| = 9.4106231E-01 *Ei/E0 = 3.3685458E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.175E+03 exceeds prior maximum 1.687E+03
automatically REFORMING stiffness matrix...
Iteration: 29 *|du|/|u| = 9.3413133E-01 *Ei/E0 = 4.6114592E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.571E+03 exceeds prior maximum 2.175E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 4.64159E-04 dt(new) = 2.15443E-04
------------------------------------------------------------
BEGIN implicit dynamics step 34 t= 3.3022E-01 06/26/25 18:13:21
============================================================
time = 3.30215E-01
current step size = 2.15443E-04
Iteration: 1 *|du|/|u| = 2.5506294E-03 *Ei/E0 = 4.8227721E-03
Iteration: 2 *|du|/|u| = 8.8180359E-03 *Ei/E0 = 1.5073962E-02
Iteration: 3 *|du|/|u| = 1.4912457E-03 *Ei/E0 = 6.7894756E-04
Equilibrium established after 3 iterations 06/26/25 18:13:21
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 2.15443E-04 dt(new) = 3.41455E-04
------------------------------------------------------------
850 t 3.3022E-01 dt 2.15E-04 write d3plot file 06/26/25 18:13:21
BEGIN implicit dynamics step 35 t= 3.3056E-01 06/26/25 18:13:21
============================================================
time = 3.30557E-01
current step size = 3.41455E-04
Iteration: 1 *|du|/|u| = 1.8673771E-02 *Ei/E0 = 6.5982117E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.243E+00 exceeds prior maximum 7.182E-01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.6873844E-02 *Ei/E0 = 6.6302037E-03
Iteration: 3 *|du|/|u| = 1.6874960E-02 *Ei/E0 = 7.0476543E-03
Iteration: 4 *|du|/|u| = 1.7042355E-02 *Ei/E0 = 7.6492492E-03
Iteration: 5 *|du|/|u| = 1.7173434E-02 *Ei/E0 = 7.9903697E-03
Iteration: 6 *|du|/|u| = 1.7276684E-02 *Ei/E0 = 8.2436443E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.314E+00 exceeds prior maximum 1.243E+00
automatically REFORMING stiffness matrix...
Iteration: 7 *|du|/|u| = 1.7581247E-02 *Ei/E0 = 8.6543449E-03
Iteration: 8 *|du|/|u| = 1.8094597E-02 *Ei/E0 = 9.7372592E-03
Iteration: 9 *|du|/|u| = 1.8549713E-02 *Ei/E0 = 1.0660165E-02
Iteration: 10 *|du|/|u| = 1.8900077E-02 *Ei/E0 = 1.1377464E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.367E+00 exceeds prior maximum 1.314E+00
automatically REFORMING stiffness matrix...
Iteration: 11 *|du|/|u| = 1.9326119E-02 *Ei/E0 = 1.2066952E-02
Iteration: 12 *|du|/|u| = 2.0333044E-02 *Ei/E0 = 1.4149311E-02
Iteration: 13 *|du|/|u| = 2.0873694E-02 *Ei/E0 = 1.5307025E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.395E+00 exceeds prior maximum 1.367E+00
automatically REFORMING stiffness matrix...
Iteration: 14 *|du|/|u| = 2.1406679E-02 *Ei/E0 = 1.6334287E-02
Iteration: 15 *|du|/|u| = 2.2888109E-02 *Ei/E0 = 1.9706000E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.424E+00 exceeds prior maximum 1.395E+00
automatically REFORMING stiffness matrix...
Iteration: 16 *|du|/|u| = 2.3528026E-02 *Ei/E0 = 2.1124510E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.453E+00 exceeds prior maximum 1.424E+00
automatically REFORMING stiffness matrix...
Iteration: 17 *|du|/|u| = 2.5568649E-02 *Ei/E0 = 2.6190347E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.566E+00 exceeds prior maximum 1.453E+00
automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 2.8066455E-02 *Ei/E0 = 3.2996089E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.612E+00 exceeds prior maximum 1.566E+00
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 3.0075879E-02 *Ei/E0 = 3.8985159E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.701E+00 exceeds prior maximum 1.612E+00
automatically REFORMING stiffness matrix...
Iteration: 20 *|du|/|u| = 3.2383234E-02 *Ei/E0 = 4.6423816E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.833E+00 exceeds prior maximum 1.701E+00
automatically REFORMING stiffness matrix...
Iteration: 21 *|du|/|u| = 3.5050442E-02 *Ei/E0 = 5.5734240E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.891E+00 exceeds prior maximum 1.833E+00
automatically REFORMING stiffness matrix...
Iteration: 22 *|du|/|u| = 3.7086738E-02 *Ei/E0 = 6.3398449E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.973E+00 exceeds prior maximum 1.891E+00
automatically REFORMING stiffness matrix...
Iteration: 23 *|du|/|u| = 3.9339995E-02 *Ei/E0 = 7.2424684E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.080E+00 exceeds prior maximum 1.973E+00
automatically REFORMING stiffness matrix...
Iteration: 24 *|du|/|u| = 4.1840709E-02 *Ei/E0 = 8.3113009E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.213E+00 exceeds prior maximum 2.080E+00
automatically REFORMING stiffness matrix...
Iteration: 25 *|du|/|u| = 4.4625890E-02 *Ei/E0 = 9.5847846E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.375E+00 exceeds prior maximum 2.213E+00
automatically REFORMING stiffness matrix...
Iteration: 26 *|du|/|u| = 4.7740987E-02 *Ei/E0 = 1.1112807E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.571E+00 exceeds prior maximum 2.375E+00
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 3.41455E-04 dt(new) = 1.58489E-04
------------------------------------------------------------
BEGIN implicit dynamics step 35 t= 3.3037E-01 06/26/25 18:13:27
============================================================
time = 3.30374E-01
current step size = 1.58489E-04
Iteration: 1 *|du|/|u| = 1.1467302E-02 *Ei/E0 = 5.9052160E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.410E-01 exceeds prior maximum 5.701E-01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 5.7541469E-03 *Ei/E0 = 2.7122085E-03
Iteration: 3 *|du|/|u| = 2.1695925E-02 *Ei/E0 = 9.3189849E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.715E+00 exceeds prior maximum 8.410E-01
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 4.7625672E-03 *Ei/E0 = 2.2409232E-03
Iteration: 5 *|du|/|u| = 2.5410288E-02 *Ei/E0 = 1.0727807E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.837E+00 exceeds prior maximum 2.715E+00
automatically REFORMING stiffness matrix...
Iteration: 6 *|du|/|u| = 5.7720173E-03 *Ei/E0 = 3.1275218E-03
Iteration: 7 *|du|/|u| = 1.7353432E-01 *Ei/E0 = 9.3740160E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.551E+00 exceeds prior maximum 3.837E+00
automatically REFORMING stiffness matrix...
Iteration: 8 *|du|/|u| = 5.7612771E-03 *Ei/E0 = 4.5993647E-03
Iteration: 9 *|du|/|u| = 6.4033748E-03 *Ei/E0 = 5.3405483E-03
Iteration: 10 *|du|/|u| = 6.5308241E-03 *Ei/E0 = 5.5653538E-03
Iteration: 11 *|du|/|u| = 6.6621104E-03 *Ei/E0 = 5.8028415E-03
Iteration: 12 *|du|/|u| = 6.7974065E-03 *Ei/E0 = 6.0538151E-03
Iteration: 13 *|du|/|u| = 6.8901153E-03 *Ei/E0 = 6.2297710E-03
Iteration: 14 *|du|/|u| = 6.9846260E-03 *Ei/E0 = 6.4124532E-03
Iteration: 15 *|du|/|u| = 7.0809692E-03 *Ei/E0 = 6.6021652E-03
Iteration: 16 *|du|/|u| = 7.1791783E-03 *Ei/E0 = 6.7992289E-03
Iteration: 17 *|du|/|u| = 7.2792899E-03 *Ei/E0 = 7.0039854E-03
Iteration: 18 *|du|/|u| = 7.3813431E-03 *Ei/E0 = 7.2167951E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 7.6429805E-03 *Ei/E0 = 7.6621766E-03
Iteration: 20 *|du|/|u| = 8.8999451E-03 *Ei/E0 = 1.0558148E-02
Iteration: 21 *|du|/|u| = 9.0512858E-03 *Ei/E0 = 1.0946082E-02
Iteration: 22 *|du|/|u| = 9.2063665E-03 *Ei/E0 = 1.1352714E-02
Iteration: 23 *|du|/|u| = 9.3652727E-03 *Ei/E0 = 1.1779132E-02
Iteration: 24 *|du|/|u| = 9.5280950E-03 *Ei/E0 = 1.2226501E-02
Iteration: 25 *|du|/|u| = 9.6949287E-03 *Ei/E0 = 1.2696065E-02
Iteration: 26 *|du|/|u| = 9.8658729E-03 *Ei/E0 = 1.3189155E-02
Iteration: 27 *|du|/|u| = 1.0041030E-02 *Ei/E0 = 1.3707195E-02
Iteration: 28 *|du|/|u| = 1.0220508E-02 *Ei/E0 = 1.4251704E-02
Iteration: 29 *|du|/|u| = 1.0404413E-02 *Ei/E0 = 1.4824310E-02
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 30 *|du|/|u| = 1.0974143E-02 *Ei/E0 = 1.6215279E-02
Iteration: 31 *|du|/|u| = 1.3442222E-02 *Ei/E0 = 2.4958694E-02
Iteration: 32 *|du|/|u| = 1.3654055E-02 *Ei/E0 = 2.5823523E-02
Iteration: 33 *|du|/|u| = 1.3870566E-02 *Ei/E0 = 2.6727072E-02
Iteration: 34 *|du|/|u| = 1.4091823E-02 *Ei/E0 = 2.7671342E-02
Iteration: 35 *|du|/|u| = 1.4317898E-02 *Ei/E0 = 2.8658452E-02
Iteration: 36 *|du|/|u| = 1.4548860E-02 *Ei/E0 = 2.9690638E-02
Iteration: 37 *|du|/|u| = 1.4784777E-02 *Ei/E0 = 3.0770265E-02
Iteration: 38 *|du|/|u| = 1.5025718E-02 *Ei/E0 = 3.1899832E-02
Iteration: 39 *|du|/|u| = 1.5271748E-02 *Ei/E0 = 3.3081979E-02
Iteration: 40 *|du|/|u| = 1.5522931E-02 *Ei/E0 = 3.4319493E-02
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 41 *|du|/|u| = 1.6690366E-02 *Ei/E0 = 3.8455072E-02
Iteration: 42 *|du|/|u| = 1.8411795E-02 *Ei/E0 = 4.7436265E-02
Iteration: 43 *|du|/|u| = 1.9762001E-02 *Ei/E0 = 5.5250379E-02
Iteration: 44 *|du|/|u| = 2.0422365E-02 *Ei/E0 = 5.9353973E-02
Iteration: 45 *|du|/|u| = 2.1115332E-02 *Ei/E0 = 6.3851134E-02
Iteration: 46 *|du|/|u| = 2.1596965E-02 *Ei/E0 = 6.7109218E-02
Iteration: 47 *|du|/|u| = 2.2092725E-02 *Ei/E0 = 7.0575459E-02
Iteration: 48 *|du|/|u| = 2.2602595E-02 *Ei/E0 = 7.4263684E-02
Iteration: 49 *|du|/|u| = 2.3126516E-02 *Ei/E0 = 7.8188619E-02
Iteration: 50 *|du|/|u| = 2.3664380E-02 *Ei/E0 = 8.2365910E-02
Iteration: 51 *|du|/|u| = 2.4216018E-02 *Ei/E0 = 8.6812142E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.929E+00 exceeds prior maximum 4.551E+00
automatically REFORMING stiffness matrix...
Iteration: 52 *|du|/|u| = 2.7072310E-02 *Ei/E0 = 1.0315084E-01
Iteration: 53 *|du|/|u| = 3.1325927E-02 *Ei/E0 = 1.4097318E-01
Iteration: 54 *|du|/|u| = 3.2875720E-02 *Ei/E0 = 1.5657430E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.954E+00 exceeds prior maximum 4.929E+00
automatically REFORMING stiffness matrix...
Iteration: 55 *|du|/|u| = 3.5919987E-02 *Ei/E0 = 1.8145337E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.146E+00 exceeds prior maximum 4.954E+00
automatically REFORMING stiffness matrix...
Iteration: 56 *|du|/|u| = 4.5334122E-02 *Ei/E0 = 2.8723075E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.420E+00 exceeds prior maximum 5.146E+00
automatically REFORMING stiffness matrix...
Iteration: 57 *|du|/|u| = 5.4958466E-02 *Ei/E0 = 4.1828672E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.641E+00 exceeds prior maximum 5.420E+00
automatically REFORMING stiffness matrix...
Iteration: 58 *|du|/|u| = 6.3986044E-02 *Ei/E0 = 5.6196679E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.116E+00 exceeds prior maximum 5.641E+00
automatically REFORMING stiffness matrix...
Iteration: 59 *|du|/|u| = 7.6030773E-02 *Ei/E0 = 7.8285388E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.428E+00 exceeds prior maximum 6.116E+00
automatically REFORMING stiffness matrix...
Iteration: 60 *|du|/|u| = 8.6768379E-02 *Ei/E0 = 1.0083126E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.920E+00 exceeds prior maximum 6.428E+00
automatically REFORMING stiffness matrix...
Iteration: 61 *|du|/|u| = 1.0036976E-01 *Ei/E0 = 1.3300054E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.223E+00 exceeds prior maximum 6.920E+00
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.58489E-04 dt(new) = 7.35642E-05
------------------------------------------------------------
BEGIN implicit dynamics step 35 t= 3.3029E-01 06/26/25 18:13:40
============================================================
time = 3.30289E-01
current step size = 7.35642E-05
Iteration: 1 *|du|/|u| = 5.7598260E-03 *Ei/E0 = 5.3683809E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.456E-01 exceeds prior maximum 5.825E-01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.2580090E-03 *Ei/E0 = 1.4896147E-03
Equilibrium established after 2 iterations 06/26/25 18:13:40
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 7.35642E-05 dt(new) = 1.16591E-04
------------------------------------------------------------
BEGIN implicit dynamics step 36 t= 3.3041E-01 06/26/25 18:13:40
============================================================
time = 3.30406E-01
current step size = 1.16591E-04
Iteration: 1 *|du|/|u| = 1.3869187E-02 *Ei/E0 = 1.2944986E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.634E-01 exceeds prior maximum 6.771E-01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 5.3063706E-03 *Ei/E0 = 2.9315886E-03
Iteration: 3 *|du|/|u| = 1.0715947E-02 *Ei/E0 = 4.6781336E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.626E-01 exceeds prior maximum 8.634E-01
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 2.2416111E-03 *Ei/E0 = 8.1168948E-04
Equilibrium established after 4 iterations 06/26/25 18:13:41
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.16591E-04 dt(new) = 1.84785E-04
------------------------------------------------------------
1548 t 3.3041E-01 dt 1.17E-04 write d3plot file 06/26/25 18:13:41
BEGIN implicit dynamics step 37 t= 3.3059E-01 06/26/25 18:13:41
============================================================
time = 3.30590E-01
current step size = 1.84785E-04
Iteration: 1 *|du|/|u| = 4.9853106E-02 *Ei/E0 = 1.0081299E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.784E+00 exceeds prior maximum 9.366E-01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 4.2483640E-02 *Ei/E0 = 9.1510004E-02
Iteration: 3 *|du|/|u| = 1.3718542E+00 *Ei/E0 = 3.1524605E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.707E+01 exceeds prior maximum 3.784E+00
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 3.8934536E-02 *Ei/E0 = 1.7295510E-01
Iteration: 5 *|du|/|u| = 4.5940647E-02 *Ei/E0 = 2.0949450E-01
Iteration: 6 *|du|/|u| = 4.6491335E-02 *Ei/E0 = 2.1765493E-01
Iteration: 7 *|du|/|u| = 4.7027741E-02 *Ei/E0 = 2.2616417E-01
Iteration: 8 *|du|/|u| = 4.7546959E-02 *Ei/E0 = 2.3503146E-01
Iteration: 9 *|du|/|u| = 4.8046269E-02 *Ei/E0 = 2.4426724E-01
Iteration: 10 *|du|/|u| = 4.8523175E-02 *Ei/E0 = 2.5388334E-01
Iteration: 11 *|du|/|u| = 4.8975456E-02 *Ei/E0 = 2.6389318E-01
Iteration: 12 *|du|/|u| = 4.9401218E-02 *Ei/E0 = 2.7431200E-01
Iteration: 13 *|du|/|u| = 4.9798931E-02 *Ei/E0 = 2.8515707E-01
Iteration: 14 *|du|/|u| = 5.0167475E-02 *Ei/E0 = 2.9644787E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 15 *|du|/|u| = 6.6100599E-02 *Ei/E0 = 4.3501785E-01
Iteration: 16 *|du|/|u| = 7.4976259E-02 *Ei/E0 = 5.8969296E-01
Iteration: 17 *|du|/|u| = 7.9901725E-02 *Ei/E0 = 6.9238381E-01
Iteration: 18 *|du|/|u| = 8.2126083E-02 *Ei/E0 = 7.4576241E-01
Iteration: 19 *|du|/|u| = 8.4308168E-02 *Ei/E0 = 8.0333225E-01
Iteration: 20 *|du|/|u| = 8.6443984E-02 *Ei/E0 = 8.6505530E-01
Iteration: 21 *|du|/|u| = 8.7792549E-02 *Ei/E0 = 9.0878600E-01
Iteration: 22 *|du|/|u| = 8.9071648E-02 *Ei/E0 = 9.5432842E-01
Iteration: 23 *|du|/|u| = 9.0268527E-02 *Ei/E0 = 1.0016447E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.715E+01 exceeds prior maximum 3.707E+01
automatically REFORMING stiffness matrix...
Iteration: 24 *|du|/|u| = 1.2187800E-01 *Ei/E0 = 1.5155009E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.869E+01 exceeds prior maximum 3.715E+01
automatically REFORMING stiffness matrix...
Iteration: 25 *|du|/|u| = 1.6812708E-01 *Ei/E0 = 3.1322458E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.465E+02 exceeds prior maximum 3.869E+01
automatically REFORMING stiffness matrix...
Iteration: 26 *|du|/|u| = 3.8813522E-01 *Ei/E0 = 2.7372769E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.723E+02 exceeds prior maximum 2.465E+02
automatically REFORMING stiffness matrix...
Iteration: 27 *|du|/|u| = 4.2647405E-01 *Ei/E0 = 7.3179957E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.005E+02 exceeds prior maximum 5.723E+02
automatically REFORMING stiffness matrix...
Iteration: 28 *|du|/|u| = 4.2313543E-01 *Ei/E0 = 1.2428465E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.244E+03 exceeds prior maximum 9.005E+02
automatically REFORMING stiffness matrix...
Iteration: 29 *|du|/|u| = 3.5868506E-01 *Ei/E0 = 1.4671307E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.554E+03 exceeds prior maximum 1.244E+03
automatically REFORMING stiffness matrix...
Iteration: 30 *|du|/|u| = 3.4981778E-01 *Ei/E0 = 1.5645591E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.619E+03 exceeds prior maximum 1.554E+03
automatically REFORMING stiffness matrix...
Iteration: 31 *|du|/|u| = 3.2251452E-01 *Ei/E0 = 1.7940285E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.750E+03 exceeds prior maximum 1.619E+03
automatically REFORMING stiffness matrix...
Iteration: 32 *|du|/|u| = 3.3217653E-01 *Ei/E0 = 2.1420837E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.028E+03 exceeds prior maximum 1.750E+03
automatically REFORMING stiffness matrix...
Iteration: 33 *|du|/|u| = 4.0564269E-01 *Ei/E0 = 2.6833478E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.348E+03 exceeds prior maximum 2.028E+03
automatically REFORMING stiffness matrix...
Iteration: 34 *|du|/|u| = 4.6870346E-01 *Ei/E0 = 4.0477329E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.864E+03 exceeds prior maximum 2.348E+03
automatically REFORMING stiffness matrix...
Iteration: 35 *|du|/|u| = 3.8057583E-01 *Ei/E0 = 5.0607717E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.451E+03 exceeds prior maximum 2.864E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.84785E-04 dt(new) = 8.57696E-05
------------------------------------------------------------
BEGIN implicit dynamics step 37 t= 3.3049E-01 06/26/25 18:13:49
============================================================
time = 3.30491E-01
current step size = 8.57696E-05
Iteration: 1 *|du|/|u| = 2.9473893E-02 *Ei/E0 = 1.0277865E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.580E+00 exceeds prior maximum 1.294E+00
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.0596548E-02 *Ei/E0 = 2.1648430E-02
Iteration: 3 *|du|/|u| = 1.7125141E-02 *Ei/E0 = 2.5711543E-02
Iteration: 4 *|du|/|u| = 5.1843513E-03 *Ei/E0 = 5.1512204E-03
Iteration: 5 *|du|/|u| = 6.2528933E-03 *Ei/E0 = 5.6958983E-03
Iteration: 6 *|du|/|u| = 3.2427460E-03 *Ei/E0 = 1.5865159E-03
Iteration: 7 *|du|/|u| = 2.5950143E-03 *Ei/E0 = 8.4715565E-04
Iteration: 8 *|du|/|u| = 2.9897601E-03 *Ei/E0 = 7.2645567E-04
Iteration: 9 *|du|/|u| = 7.2838397E-03 *Ei/E0 = 1.4555924E-03
Iteration: 10 *|du|/|u| = 1.5095734E-01 *Ei/E0 = 2.9095678E-02
Iteration: 11 *|du|/|u| = 1.5636342E-01 *Ei/E0 = 3.0561298E-02
Iteration: 12 *|du|/|u| = 1.6321491E-01 *Ei/E0 = 3.2777499E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.459E+01 exceeds prior maximum 4.580E+00
automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 6.2636738E-03 *Ei/E0 = 2.3807634E-02
Iteration: 14 *|du|/|u| = 4.0498446E-02 *Ei/E0 = 1.1205017E-01
Iteration: 15 *|du|/|u| = 4.0626341E-02 *Ei/E0 = 1.1303988E-01
Iteration: 16 *|du|/|u| = 4.0669345E-02 *Ei/E0 = 1.1336654E-01
Iteration: 17 *|du|/|u| = 4.0709416E-02 *Ei/E0 = 1.1366882E-01
Iteration: 18 *|du|/|u| = 4.0751249E-02 *Ei/E0 = 1.1398226E-01
Iteration: 19 *|du|/|u| = 4.0794847E-02 *Ei/E0 = 1.1430688E-01
Iteration: 20 *|du|/|u| = 4.0840208E-02 *Ei/E0 = 1.1464273E-01
Iteration: 21 *|du|/|u| = 4.0887334E-02 *Ei/E0 = 1.1498985E-01
Iteration: 22 *|du|/|u| = 4.0936225E-02 *Ei/E0 = 1.1534830E-01
Iteration: 23 *|du|/|u| = 4.0986882E-02 *Ei/E0 = 1.1571812E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 24 *|du|/|u| = 7.3955413E-03 *Ei/E0 = 2.1568292E-02
Iteration: 25 *|du|/|u| = 9.3490334E-03 *Ei/E0 = 3.4208740E-02
Iteration: 26 *|du|/|u| = 9.6309463E-03 *Ei/E0 = 3.6278515E-02
Iteration: 27 *|du|/|u| = 9.9263084E-03 *Ei/E0 = 3.8518171E-02
Iteration: 28 *|du|/|u| = 1.0235688E-02 *Ei/E0 = 4.0942636E-02
Iteration: 29 *|du|/|u| = 1.0559679E-02 *Ei/E0 = 4.3568330E-02
Iteration: 30 *|du|/|u| = 1.0898900E-02 *Ei/E0 = 4.6413296E-02
Iteration: 31 *|du|/|u| = 1.1253991E-02 *Ei/E0 = 4.9497354E-02
Iteration: 32 *|du|/|u| = 1.1626082E-02 *Ei/E0 = 5.2842255E-02
Iteration: 33 *|du|/|u| = 1.1885093E-02 *Ei/E0 = 5.5243617E-02
Iteration: 34 *|du|/|u| = 1.2152070E-02 *Ei/E0 = 5.7780860E-02
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 35 *|du|/|u| = 1.3373659E-02 *Ei/E0 = 6.5613064E-02
Iteration: 36 *|du|/|u| = 1.5210044E-02 *Ei/E0 = 8.4373475E-02
Iteration: 37 *|du|/|u| = 1.6681111E-02 *Ei/E0 = 1.0118625E-01
Iteration: 38 *|du|/|u| = 1.7414559E-02 *Ei/E0 = 1.1019870E-01
Iteration: 39 *|du|/|u| = 1.8191494E-02 *Ei/E0 = 1.2018405E-01
Iteration: 40 *|du|/|u| = 1.8737070E-02 *Ei/E0 = 1.2749055E-01
Iteration: 41 *|du|/|u| = 1.9302756E-02 *Ei/E0 = 1.3532010E-01
Iteration: 42 *|du|/|u| = 1.9888894E-02 *Ei/E0 = 1.4371041E-01
Iteration: 43 *|du|/|u| = 2.0495773E-02 *Ei/E0 = 1.5270148E-01
Iteration: 44 *|du|/|u| = 2.1123616E-02 *Ei/E0 = 1.6233568E-01
Iteration: 45 *|du|/|u| = 2.1772565E-02 *Ei/E0 = 1.7265766E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 46 *|du|/|u| = 2.4573554E-02 *Ei/E0 = 2.0382822E-01
Iteration: 47 *|du|/|u| = 2.9296579E-02 *Ei/E0 = 2.8743385E-01
Iteration: 48 *|du|/|u| = 3.1018794E-02 *Ei/E0 = 3.2195688E-01
Iteration: 49 *|du|/|u| = 3.2239053E-02 *Ei/E0 = 3.4782694E-01
Iteration: 50 *|du|/|u| = 3.3512993E-02 *Ei/E0 = 3.7600301E-01
Iteration: 51 *|du|/|u| = 3.4393204E-02 *Ei/E0 = 3.9628471E-01
Iteration: 52 *|du|/|u| = 3.5294561E-02 *Ei/E0 = 4.1772799E-01
Iteration: 53 *|du|/|u| = 3.6216157E-02 *Ei/E0 = 4.4038127E-01
Iteration: 54 *|du|/|u| = 3.7156885E-02 *Ei/E0 = 4.6429160E-01
Iteration: 55 *|du|/|u| = 3.8115421E-02 *Ei/E0 = 4.8950395E-01
Iteration: 56 *|du|/|u| = 3.9090209E-02 *Ei/E0 = 5.1606037E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 57 *|du|/|u| = 4.4353275E-02 *Ei/E0 = 6.1544414E-01
Iteration: 58 *|du|/|u| = 5.5202080E-02 *Ei/E0 = 9.4524049E-01
Iteration: 59 *|du|/|u| = 5.6956797E-02 *Ei/E0 = 1.0074410E+00
Iteration: 60 *|du|/|u| = 5.8746003E-02 *Ei/E0 = 1.0735847E+00
Iteration: 61 *|du|/|u| = 6.0561748E-02 *Ei/E0 = 1.1437041E+00
Iteration: 62 *|du|/|u| = 6.2405005E-02 *Ei/E0 = 1.2177839E+00
Iteration: 63 *|du|/|u| = 6.3639034E-02 *Ei/E0 = 1.2696076E+00
Iteration: 64 *|du|/|u| = 6.4872581E-02 *Ei/E0 = 1.3231394E+00
Iteration: 65 *|du|/|u| = 6.6101768E-02 *Ei/E0 = 1.3783262E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.472E+01 exceeds prior maximum 1.459E+01
automatically REFORMING stiffness matrix...
Iteration: 66 *|du|/|u| = 7.3259687E-02 *Ei/E0 = 1.6108708E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.500E+01 exceeds prior maximum 1.472E+01
automatically REFORMING stiffness matrix...
Iteration: 67 *|du|/|u| = 8.7928467E-02 *Ei/E0 = 2.2957717E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.524E+01 exceeds prior maximum 1.500E+01
automatically REFORMING stiffness matrix...
Iteration: 68 *|du|/|u| = 9.8980335E-02 *Ei/E0 = 2.9171528E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.597E+01 exceeds prior maximum 1.524E+01
automatically REFORMING stiffness matrix...
Iteration: 69 *|du|/|u| = 1.1041293E-01 *Ei/E0 = 3.6745779E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.717E+01 exceeds prior maximum 1.597E+01
automatically REFORMING stiffness matrix...
Iteration: 70 *|du|/|u| = 1.1796643E-01 *Ei/E0 = 4.5609441E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.501E+02 exceeds prior maximum 1.717E+01
automatically REFORMING stiffness matrix...
Iteration: 71 *|du|/|u| = 1.4545268E-01 *Ei/E0 = 1.2540914E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.487E+02 exceeds prior maximum 1.501E+02
automatically REFORMING stiffness matrix...
Iteration: 72 *|du|/|u| = 1.5175319E-01 *Ei/E0 = 1.7979286E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.337E+02 exceeds prior maximum 2.487E+02
automatically REFORMING stiffness matrix...
Iteration: 73 *|du|/|u| = 1.8219569E-01 *Ei/E0 = 2.8977654E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.335E+02 exceeds prior maximum 3.337E+02
automatically REFORMING stiffness matrix...
Iteration: 74 *|du|/|u| = 1.6770008E-01 *Ei/E0 = 4.8947493E+01
Iteration: 75 *|du|/|u| = 2.4636558E-01 *Ei/E0 = 5.9469108E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.377E+02 exceeds prior maximum 5.335E+02
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 8.57696E-05 dt(new) = 3.98107E-05
------------------------------------------------------------
BEGIN implicit dynamics step 37 t= 3.3045E-01 06/26/25 18:14:09
============================================================
time = 3.30445E-01
current step size = 3.98107E-05
Iteration: 1 *|du|/|u| = 1.4179938E-02 *Ei/E0 = 9.2029235E-02
Iteration: 2 *|du|/|u| = 2.1273941E-03 *Ei/E0 = 3.5118311E-03
Equilibrium established after 2 iterations 06/26/25 18:14:09
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 3.98107E-05 dt(new) = 6.30957E-05
------------------------------------------------------------
BEGIN implicit dynamics step 38 t= 3.3051E-01 06/26/25 18:14:09
============================================================
time = 3.30509E-01
current step size = 6.30957E-05
Iteration: 1 *|du|/|u| = 3.2194789E-02 *Ei/E0 = 2.0893113E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.076E+00 exceeds prior maximum 2.292E+00
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 8.4012462E-03 *Ei/E0 = 2.5667163E-02
Iteration: 3 *|du|/|u| = 9.1341514E-03 *Ei/E0 = 1.9539484E-02
Iteration: 4 *|du|/|u| = 2.3139905E-03 *Ei/E0 = 3.0323208E-03
Iteration: 5 *|du|/|u| = 1.7050128E-03 *Ei/E0 = 1.3609576E-03
Equilibrium established after 5 iterations 06/26/25 18:14:10
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 6.30957E-05 dt(new) = 1.00000E-04
------------------------------------------------------------
2768 t 3.3051E-01 dt 6.31E-05 write d3plot file 06/26/25 18:14:10
BEGIN implicit dynamics step 39 t= 3.3061E-01 06/26/25 18:14:10
============================================================
time = 3.30609E-01
current step size = 1.00000E-04
Iteration: 1 *|du|/|u| = 1.0056267E-01 *Ei/E0 = 1.0000000E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.069E+01 exceeds prior maximum 3.514E+00
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 7.7552950E-02 *Ei/E0 = 7.7235181E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.325E+01 exceeds prior maximum 2.069E+01
automatically REFORMING stiffness matrix...
Iteration: 3 *|du|/|u| = 6.4727466E-02 *Ei/E0 = 9.2292671E-01
Iteration: 4 *|du|/|u| = 7.0740318E-02 *Ei/E0 = 1.4747291E+00
Iteration: 5 *|du|/|u| = 7.1596920E-02 *Ei/E0 = 1.5504667E+00
Iteration: 6 *|du|/|u| = 7.2392960E-02 *Ei/E0 = 1.6296548E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.512E+01 exceeds prior maximum 4.325E+01
automatically REFORMING stiffness matrix...
Iteration: 7 *|du|/|u| = 1.0385066E-01 *Ei/E0 = 2.5302518E+00
Iteration: 8 *|du|/|u| = 1.1875286E-01 *Ei/E0 = 3.7517244E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.232E+02 exceeds prior maximum 4.512E+01
automatically REFORMING stiffness matrix...
Iteration: 9 *|du|/|u| = 1.9425734E-01 *Ei/E0 = 1.6603173E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.806E+02 exceeds prior maximum 2.232E+02
automatically REFORMING stiffness matrix...
Iteration: 10 *|du|/|u| = 2.0061350E-01 *Ei/E0 = 3.2839887E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.585E+02 exceeds prior maximum 3.806E+02
automatically REFORMING stiffness matrix...
Iteration: 11 *|du|/|u| = 2.0476486E-01 *Ei/E0 = 5.9250587E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.483E+02 exceeds prior maximum 6.585E+02
automatically REFORMING stiffness matrix...
Iteration: 12 *|du|/|u| = 1.6116562E-01 *Ei/E0 = 6.2245871E+01
Iteration: 13 *|du|/|u| = 1.6429751E-01 *Ei/E0 = 5.5392733E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.328E+03 exceeds prior maximum 8.483E+02
automatically REFORMING stiffness matrix...
Iteration: 14 *|du|/|u| = 2.5345005E-01 *Ei/E0 = 7.7532666E+01
Iteration: 15 *|du|/|u| = 2.1837102E-01 *Ei/E0 = 9.1512460E+01
Iteration: 16 *|du|/|u| = 2.5859260E-01 *Ei/E0 = 1.2455967E+02
Iteration: 17 *|du|/|u| = 2.0119846E-01 *Ei/E0 = 6.7337916E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.366E+03 exceeds prior maximum 1.328E+03
automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 1.5519254E-01 *Ei/E0 = 9.7819720E+01
Iteration: 19 *|du|/|u| = 2.5472819E-01 *Ei/E0 = 1.3375003E+02
Iteration: 20 *|du|/|u| = 1.9732739E-01 *Ei/E0 = 1.1201029E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.411E+03 exceeds prior maximum 1.366E+03
automatically REFORMING stiffness matrix...
Iteration: 21 *|du|/|u| = 2.1196608E-01 *Ei/E0 = 1.2979889E+02
Iteration: 22 *|du|/|u| = 2.2581910E-01 *Ei/E0 = 1.6950534E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.593E+03 exceeds prior maximum 1.411E+03
automatically REFORMING stiffness matrix...
Iteration: 23 *|du|/|u| = 1.6059858E-01 *Ei/E0 = 1.6811772E+02
Iteration: 24 *|du|/|u| = 1.5416797E-01 *Ei/E0 = 1.7650384E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.661E+03 exceeds prior maximum 1.593E+03
automatically REFORMING stiffness matrix...
Iteration: 25 *|du|/|u| = 1.5210574E-01 *Ei/E0 = 1.2978889E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.706E+03 exceeds prior maximum 1.661E+03
automatically REFORMING stiffness matrix...
Iteration: 26 *|du|/|u| = 1.1297149E-01 *Ei/E0 = 2.1857683E+02
Iteration: 27 *|du|/|u| = 1.3766731E-01 *Ei/E0 = 1.4980341E+02
Iteration: 28 *|du|/|u| = 1.9841946E-01 *Ei/E0 = 1.2924757E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.778E+03 exceeds prior maximum 1.706E+03
automatically REFORMING stiffness matrix...
Iteration: 29 *|du|/|u| = 1.3205847E-01 *Ei/E0 = 1.3799567E+02
Iteration: 30 *|du|/|u| = 1.6258970E-01 *Ei/E0 = 1.7855453E+02
Iteration: 31 *|du|/|u| = 2.0534860E-01 *Ei/E0 = 2.3055355E+02
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.932E+03 exceeds prior maximum 1.778E+03
automatically REFORMING stiffness matrix...
Iteration: 32 *|du|/|u| = 1.9581983E-01 *Ei/E0 = 3.0505873E+02
Iteration: 33 *|du|/|u| = 1.6333406E-01 *Ei/E0 = 2.8631576E+02
Iteration: 34 *|du|/|u| = 1.6720505E-01 *Ei/E0 = 2.5933145E+02
Iteration: 35 *|du|/|u| = 1.5371950E-01 *Ei/E0 = 1.4629704E+02
Iteration: 36 *|du|/|u| = 1.0864626E-01 *Ei/E0 = 8.2472200E+01
Iteration: 37 *|du|/|u| = 2.1037195E-01 *Ei/E0 = 1.6989666E+02
Iteration: 38 *|du|/|u| = 1.0693429E-01 *Ei/E0 = 9.8778972E+01
Iteration: 39 *|du|/|u| = 9.6314808E-02 *Ei/E0 = 7.5031403E+01
Iteration: 40 *|du|/|u| = 7.5864873E-02 *Ei/E0 = 2.6828472E+01
Iteration: 41 *|du|/|u| = 8.5606191E-02 *Ei/E0 = 5.8162648E+01
Iteration: 42 *|du|/|u| = 5.9165866E-02 *Ei/E0 = 3.7949240E+01
REFORMATION LIMIT reached, nonlinear equilibrium search aborted...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.00000E-04 dt(new) = 4.64159E-05
------------------------------------------------------------
BEGIN implicit dynamics step 39 t= 3.3055E-01 06/26/25 18:14:16
============================================================
time = 3.30555E-01
current step size = 4.64159E-05
Iteration: 1 *|du|/|u| = 5.1216516E-02 *Ei/E0 = 9.5012830E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.826E+01 exceeds prior maximum 5.980E+00
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.2431795E-02 *Ei/E0 = 1.3886825E-01
Iteration: 3 *|du|/|u| = 1.6492244E-02 *Ei/E0 = 1.3053039E-01
Iteration: 4 *|du|/|u| = 4.0187781E-03 *Ei/E0 = 1.0719302E-02
Iteration: 5 *|du|/|u| = 1.6979091E-03 *Ei/E0 = 2.0379940E-03
Equilibrium established after 5 iterations 06/26/25 18:14:16
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 4.64159E-05 dt(new) = 7.35642E-05
------------------------------------------------------------
BEGIN implicit dynamics step 40 t= 3.3063E-01 06/26/25 18:14:16
============================================================
time = 3.30628E-01
current step size = 7.35642E-05
Iteration: 1 *|du|/|u| = 1.8624506E-01 *Ei/E0 = 1.0000000E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.465E+01 exceeds prior maximum 8.859E+00
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.5650652E-01 *Ei/E0 = 1.1387277E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.244E+02 exceeds prior maximum 8.465E+01
automatically REFORMING stiffness matrix...
Iteration: 3 *|du|/|u| = 1.5393036E-01 *Ei/E0 = 2.4572295E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.362E+02 exceeds prior maximum 2.244E+02
automatically REFORMING stiffness matrix...
Iteration: 4 *|du|/|u| = 1.3220985E-01 *Ei/E0 = 2.7149586E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.486E+02 exceeds prior maximum 2.362E+02
automatically REFORMING stiffness matrix...
Iteration: 5 *|du|/|u| = 1.2503595E-01 *Ei/E0 = 2.6850168E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.686E+02 exceeds prior maximum 2.486E+02
automatically REFORMING stiffness matrix...
Iteration: 6 *|du|/|u| = 1.1270762E-01 *Ei/E0 = 2.8658909E+00
Iteration: 7 *|du|/|u| = 2.7382037E-01 *Ei/E0 = 6.0793698E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.272E+02 exceeds prior maximum 2.686E+02
automatically REFORMING stiffness matrix...
Iteration: 8 *|du|/|u| = 9.8045008E-02 *Ei/E0 = 4.5487414E+00
Iteration: 9 *|du|/|u| = 2.8132381E-01 *Ei/E0 = 8.2781380E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.092E+02 exceeds prior maximum 5.272E+02
automatically REFORMING stiffness matrix...
Iteration: 10 *|du|/|u| = 1.1467341E-01 *Ei/E0 = 7.1724745E+00
Iteration: 11 *|du|/|u| = 2.0895065E-01 *Ei/E0 = 1.0107862E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.068E+02 exceeds prior maximum 7.092E+02
automatically REFORMING stiffness matrix...
Iteration: 12 *|du|/|u| = 9.1454244E-02 *Ei/E0 = 7.5027625E+00
Iteration: 13 *|du|/|u| = 1.7987275E-01 *Ei/E0 = 7.7287405E+00
Iteration: 14 *|du|/|u| = 1.8220161E-01 *Ei/E0 = 8.4985607E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.046E+03 exceeds prior maximum 8.068E+02
automatically REFORMING stiffness matrix...
Iteration: 15 *|du|/|u| = 1.3974862E-01 *Ei/E0 = 1.3238575E+01
Iteration: 16 *|du|/|u| = 1.8570063E-01 *Ei/E0 = 1.6810336E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.135E+03 exceeds prior maximum 1.046E+03
automatically REFORMING stiffness matrix...
Iteration: 17 *|du|/|u| = 1.4428269E-01 *Ei/E0 = 1.9155571E+01
Iteration: 18 *|du|/|u| = 1.6294339E-01 *Ei/E0 = 2.1807549E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.227E+03 exceeds prior maximum 1.135E+03
automatically REFORMING stiffness matrix...
Iteration: 19 *|du|/|u| = 1.0692131E-01 *Ei/E0 = 1.8959335E+01
Iteration: 20 *|du|/|u| = 1.5929553E-01 *Ei/E0 = 1.9687654E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.526E+03 exceeds prior maximum 1.227E+03
automatically REFORMING stiffness matrix...
Iteration: 21 *|du|/|u| = 1.0045599E-01 *Ei/E0 = 1.9112296E+01
Iteration: 22 *|du|/|u| = 2.0385166E-01 *Ei/E0 = 2.1856387E+01
Iteration: 23 *|du|/|u| = 1.9623100E-01 *Ei/E0 = 1.7995161E+01
Iteration: 24 *|du|/|u| = 7.7379845E-02 *Ei/E0 = 1.2537624E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.531E+03 exceeds prior maximum 1.526E+03
automatically REFORMING stiffness matrix...
Iteration: 25 *|du|/|u| = 8.6726371E-02 *Ei/E0 = 1.1454789E+01
Iteration: 26 *|du|/|u| = 9.5329751E-02 *Ei/E0 = 1.2382331E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.140E+03 exceeds prior maximum 1.531E+03
automatically REFORMING stiffness matrix...
Iteration: 27 *|du|/|u| = 9.2505213E-02 *Ei/E0 = 2.2067972E+01
Iteration: 28 *|du|/|u| = 1.0561716E-01 *Ei/E0 = 1.5458560E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.466E+03 exceeds prior maximum 2.140E+03
automatically REFORMING stiffness matrix...
Iteration: 29 *|du|/|u| = 9.7230669E-02 *Ei/E0 = 1.9875799E+01
Iteration: 30 *|du|/|u| = 1.1452134E-01 *Ei/E0 = 3.2673424E+01
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.136E+03 exceeds prior maximum 2.466E+03
automatically REFORMING stiffness matrix...
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 7.35642E-05 dt(new) = 3.41455E-05
------------------------------------------------------------
BEGIN implicit dynamics step 40 t= 3.3059E-01 06/26/25 18:14:20
============================================================
time = 3.30589E-01
current step size = 3.41455E-05
Iteration: 1 *|du|/|u| = 8.5749736E-02 *Ei/E0 = 1.0000000E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.029E+01 exceeds prior maximum 1.544E+01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.5378752E-02 *Ei/E0 = 2.4204414E-01
Iteration: 3 *|du|/|u| = 3.0649585E-02 *Ei/E0 = 1.5717423E-01
Iteration: 4 *|du|/|u| = 3.1248205E-03 *Ei/E0 = 5.6416087E-03
Iteration: 5 *|du|/|u| = 1.6944702E-03 *Ei/E0 = 5.4278995E-04
Equilibrium established after 5 iterations 06/26/25 18:14:21
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 3.41455E-05 dt(new) = 5.41170E-05
------------------------------------------------------------
BEGIN implicit dynamics step 41 t= 3.3064E-01 06/26/25 18:14:21
============================================================
time = 3.30643E-01
current step size = 5.41170E-05
Iteration: 1 *|du|/|u| = 3.7759663E-01 *Ei/E0 = 4.3230497E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.076E+02 exceeds prior maximum 2.425E+01
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.8553931E-01 *Ei/E0 = 3.9205244E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 4.880E+02 exceeds prior maximum 1.076E+02
automatically REFORMING stiffness matrix...
Iteration: 3 *|du|/|u| = 1.3166834E-01 *Ei/E0 = 3.0847975E-01
Iteration: 4 *|du|/|u| = 1.0357264E-01 *Ei/E0 = 1.4598251E-01
Iteration: 5 *|du|/|u| = 8.7666356E-02 *Ei/E0 = 1.1601209E-01
Iteration: 6 *|du|/|u| = 6.5629611E-02 *Ei/E0 = 7.5992667E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.805E+02 exceeds prior maximum 4.880E+02
automatically REFORMING stiffness matrix...
Iteration: 7 *|du|/|u| = 5.1922639E-02 *Ei/E0 = 9.0541717E-02
Iteration: 8 *|du|/|u| = 5.0513383E-02 *Ei/E0 = 2.5604167E-02
Iteration: 9 *|du|/|u| = 1.5435804E-01 *Ei/E0 = 8.7139830E-02
Iteration: 10 *|du|/|u| = 5.6558257E-02 *Ei/E0 = 5.7071898E-02
Iteration: 11 *|du|/|u| = 7.5095183E-02 *Ei/E0 = 6.4686323E-02
Iteration: 12 *|du|/|u| = 6.9288838E-02 *Ei/E0 = 9.0706738E-02
Iteration: 13 *|du|/|u| = 8.0484567E-02 *Ei/E0 = 6.4989415E-03
Iteration: 14 *|du|/|u| = 6.2015453E-02 *Ei/E0 = 4.6894420E-02
Iteration: 15 *|du|/|u| = 5.0366001E-02 *Ei/E0 = 3.1641949E-02
Iteration: 16 *|du|/|u| = 3.9515748E-02 *Ei/E0 = 3.5230578E-02
Iteration: 17 *|du|/|u| = 7.0182655E-02 *Ei/E0 = 7.1975999E-02
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 18 *|du|/|u| = 4.1952066E-02 *Ei/E0 = 6.5816027E-02
Iteration: 19 *|du|/|u| = 7.7990877E-02 *Ei/E0 = 6.0404645E-02
Iteration: 20 *|du|/|u| = 1.2655399E-01 *Ei/E0 = 1.0582375E-01
Iteration: 21 *|du|/|u| = 5.1821584E-02 *Ei/E0 = 7.3589660E-02
Iteration: 22 *|du|/|u| = 5.7362195E-02 *Ei/E0 = 5.1072705E-02
Iteration: 23 *|du|/|u| = 6.2484811E-02 *Ei/E0 = 3.7533604E-02
Iteration: 24 *|du|/|u| = 9.3021823E-02 *Ei/E0 = 6.7109665E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.662E+02 exceeds prior maximum 5.805E+02
automatically REFORMING stiffness matrix...
Iteration: 25 *|du|/|u| = 4.9526061E-02 *Ei/E0 = 1.8122174E-01
Iteration: 26 *|du|/|u| = 6.9223364E-02 *Ei/E0 = 8.6548647E-02
Iteration: 27 *|du|/|u| = 1.6368177E-01 *Ei/E0 = 2.2847555E-01
Iteration: 28 *|du|/|u| = 5.9029974E-02 *Ei/E0 = 1.2988370E-01
Iteration: 29 *|du|/|u| = 7.8131617E-02 *Ei/E0 = 1.8011884E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.141E+02 exceeds prior maximum 8.662E+02
automatically REFORMING stiffness matrix...
Iteration: 30 *|du|/|u| = 4.7518707E-02 *Ei/E0 = 1.8906349E-01
Iteration: 31 *|du|/|u| = 5.8700427E-02 *Ei/E0 = 8.7337209E-02
Iteration: 32 *|du|/|u| = 1.3721059E-01 *Ei/E0 = 2.3357502E-01
Iteration: 33 *|du|/|u| = 5.3583325E-02 *Ei/E0 = 1.4673074E-01
Iteration: 34 *|du|/|u| = 6.6378950E-02 *Ei/E0 = 1.5181070E-01
Iteration: 35 *|du|/|u| = 5.1760656E-02 *Ei/E0 = 1.6729424E-01
Iteration: 36 *|du|/|u| = 6.4033232E-02 *Ei/E0 = 8.4312243E-02
Iteration: 37 *|du|/|u| = 5.2097560E-02 *Ei/E0 = 9.6805532E-02
Iteration: 38 *|du|/|u| = 5.3975960E-02 *Ei/E0 = 1.1090321E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.008E+03 exceeds prior maximum 9.141E+02
automatically REFORMING stiffness matrix...
Iteration: 39 *|du|/|u| = 4.6116500E-02 *Ei/E0 = 1.9714439E-01
Iteration: 40 *|du|/|u| = 8.7149515E-02 *Ei/E0 = 2.2585249E-01
Iteration: 41 *|du|/|u| = 5.8139773E-02 *Ei/E0 = 2.4764779E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.035E+03 exceeds prior maximum 1.008E+03
automatically REFORMING stiffness matrix...
Iteration: 42 *|du|/|u| = 3.1565394E-02 *Ei/E0 = 2.0731393E-01
Iteration: 43 *|du|/|u| = 2.6047629E-02 *Ei/E0 = 4.0876279E-02
Iteration: 44 *|du|/|u| = 5.1220481E-02 *Ei/E0 = 6.8774175E-02
Iteration: 45 *|du|/|u| = 9.2157761E-03 *Ei/E0 = 3.7973479E-02
Iteration: 46 *|du|/|u| = 3.2117639E-02 *Ei/E0 = 4.3307020E-02
Iteration: 47 *|du|/|u| = 5.0011190E-02 *Ei/E0 = 3.0565073E-02
Iteration: 48 *|du|/|u| = 3.4071014E-02 *Ei/E0 = 1.1782000E-01
Iteration: 49 *|du|/|u| = 1.4467685E-01 *Ei/E0 = 6.5796707E-01
Iteration: 50 *|du|/|u| = 6.2007450E-02 *Ei/E0 = 3.8017141E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.117E+03 exceeds prior maximum 1.035E+03
automatically REFORMING stiffness matrix...
Iteration: 51 *|du|/|u| = 6.8740103E-02 *Ei/E0 = 7.1848484E-01
Iteration: 52 *|du|/|u| = 4.1760761E-02 *Ei/E0 = 3.4946166E-01
Iteration: 53 *|du|/|u| = 3.8138897E-02 *Ei/E0 = 1.1445175E-01
Iteration: 54 *|du|/|u| = 3.2053498E-02 *Ei/E0 = 9.8327133E-02
Iteration: 55 *|du|/|u| = 2.1092147E-02 *Ei/E0 = 9.3204451E-02
Iteration: 56 *|du|/|u| = 1.7611896E-02 *Ei/E0 = 9.2911987E-02
Iteration: 57 *|du|/|u| = 1.7608738E-02 *Ei/E0 = 8.4104163E-02
Iteration: 58 *|du|/|u| = 1.9137440E-02 *Ei/E0 = 6.2734396E-02
Iteration: 59 *|du|/|u| = 2.3611547E-02 *Ei/E0 = 7.0724721E-02
Iteration: 60 *|du|/|u| = 1.6323993E-02 *Ei/E0 = 5.2164092E-02
Iteration: 61 *|du|/|u| = 1.6128040E-02 *Ei/E0 = 2.6794922E-02
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 62 *|du|/|u| = 1.4295435E-02 *Ei/E0 = 3.8368083E-02
Iteration: 63 *|du|/|u| = 2.5048380E-02 *Ei/E0 = 4.1915081E-02
Iteration: 64 *|du|/|u| = 6.8432269E-02 *Ei/E0 = 1.1556482E-01
Iteration: 65 *|du|/|u| = 2.7198329E-02 *Ei/E0 = 5.7240639E-02
Iteration: 66 *|du|/|u| = 2.2989672E-02 *Ei/E0 = 7.7427832E-02
Iteration: 67 *|du|/|u| = 2.1385903E-02 *Ei/E0 = 5.4022617E-02
Iteration: 68 *|du|/|u| = 2.4716490E-02 *Ei/E0 = 7.4838189E-02
Iteration: 69 *|du|/|u| = 2.5857815E-02 *Ei/E0 = 1.1044298E-01
Iteration: 70 *|du|/|u| = 2.7985925E-02 *Ei/E0 = 1.0264680E-01
Iteration: 71 *|du|/|u| = 3.1547495E-02 *Ei/E0 = 1.2630375E-01
Iteration: 72 *|du|/|u| = 6.4631734E-02 *Ei/E0 = 1.7392665E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 73 *|du|/|u| = 2.9164522E-02 *Ei/E0 = 1.9548917E-01
Iteration: 74 *|du|/|u| = 4.2012755E-02 *Ei/E0 = 1.9905666E-01
Iteration: 75 *|du|/|u| = 1.5839583E-02 *Ei/E0 = 1.9913493E-01
Iteration: 76 *|du|/|u| = 1.7516054E-02 *Ei/E0 = 9.4807944E-02
Iteration: 77 *|du|/|u| = 2.7140610E-02 *Ei/E0 = 1.1169044E-01
Iteration: 78 *|du|/|u| = 3.9010771E-02 *Ei/E0 = 9.6473254E-02
Iteration: 79 *|du|/|u| = 2.0818514E-02 *Ei/E0 = 4.6613551E-02
Iteration: 80 *|du|/|u| = 3.2214159E-02 *Ei/E0 = 7.0203981E-02
Iteration: 81 *|du|/|u| = 3.0814587E-02 *Ei/E0 = 8.0985119E-02
Iteration: 82 *|du|/|u| = 4.1907158E-02 *Ei/E0 = 1.0554148E-01
Iteration: 83 *|du|/|u| = 5.6088282E-02 *Ei/E0 = 1.7025006E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 84 *|du|/|u| = 2.3367246E-02 *Ei/E0 = 1.2326927E-01
Iteration: 85 *|du|/|u| = 3.1231473E-02 *Ei/E0 = 1.2806858E-01
Iteration: 86 *|du|/|u| = 4.2506367E-02 *Ei/E0 = 2.3411383E-01
Iteration: 87 *|du|/|u| = 3.2193200E-02 *Ei/E0 = 1.8761819E-01
Iteration: 88 *|du|/|u| = 7.0306721E-02 *Ei/E0 = 2.7693479E-01
Iteration: 89 *|du|/|u| = 3.5957550E-02 *Ei/E0 = 1.5643834E-01
Iteration: 90 *|du|/|u| = 2.5581154E-02 *Ei/E0 = 2.2044223E-01
Iteration: 91 *|du|/|u| = 5.8040705E-02 *Ei/E0 = 3.0424117E-01
Iteration: 92 *|du|/|u| = 1.2448414E-01 *Ei/E0 = 2.8299847E-01
Iteration: 93 *|du|/|u| = 7.8402700E-02 *Ei/E0 = 3.9490801E-01
Iteration: 94 *|du|/|u| = 3.9079476E-02 *Ei/E0 = 2.2328772E-01
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 95 *|du|/|u| = 2.7925526E-02 *Ei/E0 = 1.9934356E-01
Iteration: 96 *|du|/|u| = 3.6818166E-02 *Ei/E0 = 1.7697570E-01
Iteration: 97 *|du|/|u| = 1.4576526E-02 *Ei/E0 = 1.8115733E-01
Iteration: 98 *|du|/|u| = 1.2017298E-02 *Ei/E0 = 5.9035330E-02
Iteration: 99 *|du|/|u| = 2.7938695E-02 *Ei/E0 = 5.7932040E-02
Iteration: 100 *|du|/|u| = 2.5842112E-02 *Ei/E0 = 7.1268016E-02
Iteration: 101 *|du|/|u| = 1.6767116E-02 *Ei/E0 = 5.6357593E-02
Iteration: 102 *|du|/|u| = 2.0184830E-02 *Ei/E0 = 5.9846591E-02
Iteration: 103 *|du|/|u| = 1.4347433E-02 *Ei/E0 = 7.7375191E-02
Iteration: 104 *|du|/|u| = 1.7328967E-02 *Ei/E0 = 9.1891237E-02
Iteration: 105 *|du|/|u| = 1.7008617E-02 *Ei/E0 = 3.3341394E-02
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 106 *|du|/|u| = 1.4981128E-02 *Ei/E0 = 7.9728547E-02
Iteration: 107 *|du|/|u| = 2.1775681E-02 *Ei/E0 = 4.4051998E-02
Iteration: 108 *|du|/|u| = 2.2975216E-02 *Ei/E0 = 4.4180223E-02
Iteration: 109 *|du|/|u| = 8.1499833E-03 *Ei/E0 = 3.6647427E-02
Iteration: 110 *|du|/|u| = 8.4475503E-03 *Ei/E0 = 1.2594696E-02
Iteration: 111 *|du|/|u| = 1.4253906E-02 *Ei/E0 = 1.6260169E-02
Iteration: 112 *|du|/|u| = 5.5084596E-03 *Ei/E0 = 1.1299559E-02
Iteration: 113 *|du|/|u| = 1.3471666E-02 *Ei/E0 = 1.3205368E-02
Iteration: 114 *|du|/|u| = 7.6556141E-03 *Ei/E0 = 4.1481944E-03
Iteration: 115 *|du|/|u| = 3.5391294E-03 *Ei/E0 = 6.0675009E-03
Iteration: 116 *|du|/|u| = 4.2189945E-03 *Ei/E0 = 2.9646246E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 117 *|du|/|u| = 2.5348594E-03 *Ei/E0 = 2.5443681E-03
Iteration: 118 *|du|/|u| = 2.2076161E-03 *Ei/E0 = 7.3711574E-04
Iteration: 119 *|du|/|u| = 3.9411083E-03 *Ei/E0 = 8.8097593E-04
Iteration: 120 *|du|/|u| = 1.7337072E-03 *Ei/E0 = 2.8429229E-04
Iteration: 121 *|du|/|u| = 1.6540601E-03 *Ei/E0 = 1.9926971E-04
Iteration: 122 *|du|/|u| = 8.9458148E-04 *Ei/E0 = 5.2891454E-05
Equilibrium established after 122 iterations 06/26/25 18:14:29
------------------------------------------------------------
Converged in more than ITEOPT+ITEWIN= 16 iterations,
automatic time step size DECREASE:
dt(old) = 5.41170E-05 dt(new) = 2.71227E-05
------------------------------------------------------------
3313 t 3.3064E-01 dt 5.41E-05 write d3plot file 06/26/25 18:14:29
BEGIN implicit dynamics step 42 t= 3.3067E-01 06/26/25 18:14:29
============================================================
time = 3.30670E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 5.2078008E-01 *Ei/E0 = 6.2865069E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.907E+03 exceeds prior maximum 1.184E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.4814351E-01 *Ei/E0 = 1.5593976E-01
Iteration: 3 *|du|/|u| = 1.1341446E-02 *Ei/E0 = 4.1854405E-03
Iteration: 4 *|du|/|u| = 1.0523309E-02 *Ei/E0 = 9.5580348E-04
Iteration: 5 *|du|/|u| = 1.9543303E-02 *Ei/E0 = 2.1180834E-03
Iteration: 6 *|du|/|u| = 2.7215177E-02 *Ei/E0 = 1.4838715E-03
Iteration: 7 *|du|/|u| = 1.1881788E-02 *Ei/E0 = 1.3305542E-03
Iteration: 8 *|du|/|u| = 2.9005662E-02 *Ei/E0 = 4.2178486E-03
Iteration: 9 *|du|/|u| = 3.9712987E-02 *Ei/E0 = 4.9085636E-03
Iteration: 10 *|du|/|u| = 2.3998149E-02 *Ei/E0 = 5.1678398E-03
Iteration: 11 *|du|/|u| = 1.7213378E-02 *Ei/E0 = 3.3070661E-03
Iteration: 12 *|du|/|u| = 2.6547841E-02 *Ei/E0 = 1.6024767E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.0647798E-02 *Ei/E0 = 1.3405903E-03
Iteration: 14 *|du|/|u| = 8.6984370E-03 *Ei/E0 = 4.9574499E-04
Iteration: 15 *|du|/|u| = 2.6223777E-03 *Ei/E0 = 1.0757575E-04
Iteration: 16 *|du|/|u| = 1.5095400E-03 *Ei/E0 = 2.1914621E-05
Iteration: 17 *|du|/|u| = 2.4535251E-03 *Ei/E0 = 2.2453262E-05
Iteration: 18 *|du|/|u| = 1.0019443E-02 *Ei/E0 = 8.4861272E-05
Iteration: 19 *|du|/|u| = 4.2012610E-03 *Ei/E0 = 6.7565745E-05
Iteration: 20 *|du|/|u| = 5.2825008E-03 *Ei/E0 = 1.8756561E-04
Iteration: 21 *|du|/|u| = 2.1204513E-03 *Ei/E0 = 8.8270372E-05
Iteration: 22 *|du|/|u| = 4.0506240E-03 *Ei/E0 = 5.0047466E-05
Iteration: 23 *|du|/|u| = 2.6541686E-03 *Ei/E0 = 2.5555865E-05
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 24 *|du|/|u| = 8.1784173E-04 *Ei/E0 = 1.2405934E-05
Equilibrium established after 24 iterations 06/26/25 18:14:31
------------------------------------------------------------
Converged in more than ITEOPT+ITEWIN= 16 iterations,
automatic time step size DECREASE:
dt(old) = 2.71227E-05 dt(new) = 1.35936E-05
------------------------------------------------------------
BEGIN implicit dynamics step 43 t= 3.3068E-01 06/26/25 18:14:31
============================================================
time = 3.30684E-01
current step size = 1.35936E-05
Iteration: 1 *|du|/|u| = 2.1540998E-01 *Ei/E0 = 8.2427044E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.315E+03 exceeds prior maximum 2.454E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.5005701E-02 *Ei/E0 = 1.1779386E-01
Iteration: 3 *|du|/|u| = 2.1166786E-03 *Ei/E0 = 5.3249323E-04
Iteration: 4 *|du|/|u| = 7.6911277E-04 *Ei/E0 = 3.5002887E-05
Equilibrium established after 4 iterations 06/26/25 18:14:32
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.35936E-05 dt(new) = 2.15443E-05
------------------------------------------------------------
BEGIN implicit dynamics step 44 t= 3.3071E-01 06/26/25 18:14:32
============================================================
time = 3.30705E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 2.2598847E-01 *Ei/E0 = 6.1943297E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.199E+03 exceeds prior maximum 1.694E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.4911353E-02 *Ei/E0 = 9.8383864E-02
Iteration: 3 *|du|/|u| = 1.2989542E-02 *Ei/E0 = 5.8103914E-03
Iteration: 4 *|du|/|u| = 1.0598297E-02 *Ei/E0 = 3.3091154E-03
Iteration: 5 *|du|/|u| = 3.6924789E-03 *Ei/E0 = 5.7107178E-04
Iteration: 6 *|du|/|u| = 3.2380629E-03 *Ei/E0 = 3.6904286E-04
Iteration: 7 *|du|/|u| = 2.1434657E-03 *Ei/E0 = 1.4274259E-04
Iteration: 8 *|du|/|u| = 1.5646793E-03 *Ei/E0 = 1.0356345E-04
Iteration: 9 *|du|/|u| = 1.3631394E-03 *Ei/E0 = 4.2018964E-05
Iteration: 10 *|du|/|u| = 9.8768868E-04 *Ei/E0 = 3.4449806E-05
Equilibrium established after 10 iterations 06/26/25 18:14:32
3386 t 3.3071E-01 dt 2.15E-05 write d3plot file 06/26/25 18:14:32
BEGIN implicit dynamics step 45 t= 3.3073E-01 06/26/25 18:14:32
============================================================
time = 3.30727E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.7882454E-01 *Ei/E0 = 5.5325710E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.451E+04 exceeds prior maximum 1.713E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.6018606E-02 *Ei/E0 = 3.0697374E-01
Iteration: 3 *|du|/|u| = 7.9115880E-03 *Ei/E0 = 3.9105403E-03
Iteration: 4 *|du|/|u| = 5.2978259E-03 *Ei/E0 = 1.3365525E-03
Iteration: 5 *|du|/|u| = 2.0391305E-03 *Ei/E0 = 2.1082555E-04
Iteration: 6 *|du|/|u| = 2.1166118E-03 *Ei/E0 = 1.2625387E-04
Iteration: 7 *|du|/|u| = 1.1211744E-03 *Ei/E0 = 4.8845657E-05
Iteration: 8 *|du|/|u| = 8.7489101E-04 *Ei/E0 = 1.7297068E-05
Equilibrium established after 8 iterations 06/26/25 18:14:33
BEGIN implicit dynamics step 46 t= 3.3075E-01 06/26/25 18:14:33
============================================================
time = 3.30749E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.4410747E-01 *Ei/E0 = 4.8680355E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.431E+04 exceeds prior maximum 1.633E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.0877734E-02 *Ei/E0 = 2.4225101E-01
Iteration: 3 *|du|/|u| = 6.8001893E-03 *Ei/E0 = 3.3333816E-03
Iteration: 4 *|du|/|u| = 5.5331933E-03 *Ei/E0 = 1.6128757E-03
Iteration: 5 *|du|/|u| = 1.6464052E-03 *Ei/E0 = 2.3393101E-04
Iteration: 6 *|du|/|u| = 1.4324423E-03 *Ei/E0 = 1.2309335E-04
Iteration: 7 *|du|/|u| = 8.1199970E-04 *Ei/E0 = 6.0650430E-05
Equilibrium established after 7 iterations 06/26/25 18:14:33
BEGIN implicit dynamics step 47 t= 3.3077E-01 06/26/25 18:14:33
============================================================
time = 3.30770E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.3583419E-01 *Ei/E0 = 5.4836446E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.472E+04 exceeds prior maximum 1.820E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.1025902E-02 *Ei/E0 = 2.7664073E-01
Iteration: 3 *|du|/|u| = 6.5143757E-03 *Ei/E0 = 4.3927016E-03
Iteration: 4 *|du|/|u| = 5.3063364E-03 *Ei/E0 = 1.9426539E-03
Iteration: 5 *|du|/|u| = 2.2755532E-03 *Ei/E0 = 7.1360996E-04
Iteration: 6 *|du|/|u| = 1.0630725E-03 *Ei/E0 = 2.7005415E-04
Iteration: 7 *|du|/|u| = 8.0205458E-04 *Ei/E0 = 9.3470768E-05
Equilibrium established after 7 iterations 06/26/25 18:14:34
BEGIN implicit dynamics step 48 t= 3.3079E-01 06/26/25 18:14:34
============================================================
time = 3.30792E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.3747473E-01 *Ei/E0 = 6.7836336E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.463E+04 exceeds prior maximum 1.914E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.4533645E-02 *Ei/E0 = 2.8940348E-01
Iteration: 3 *|du|/|u| = 3.9807379E-03 *Ei/E0 = 2.2758281E-03
Iteration: 4 *|du|/|u| = 3.6170835E-03 *Ei/E0 = 8.6397190E-04
Iteration: 5 *|du|/|u| = 2.3187483E-03 *Ei/E0 = 4.6017267E-04
Iteration: 6 *|du|/|u| = 4.3012100E-03 *Ei/E0 = 5.3952256E-04
Iteration: 7 *|du|/|u| = 4.3900202E-03 *Ei/E0 = 9.8808672E-04
Iteration: 8 *|du|/|u| = 2.5861305E-03 *Ei/E0 = 9.2733975E-04
Iteration: 9 *|du|/|u| = 5.9091694E-03 *Ei/E0 = 5.6364856E-04
Iteration: 10 *|du|/|u| = 4.2039783E-03 *Ei/E0 = 1.5331476E-03
Iteration: 11 *|du|/|u| = 2.7127059E-03 *Ei/E0 = 7.4733241E-04
Iteration: 12 *|du|/|u| = 1.8829100E-03 *Ei/E0 = 6.7733205E-04
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.2654134E-03 *Ei/E0 = 5.1547479E-04
Iteration: 14 *|du|/|u| = 9.6688766E-04 *Ei/E0 = 8.6293880E-05
Equilibrium established after 14 iterations 06/26/25 18:14:35
BEGIN implicit dynamics step 49 t= 3.3081E-01 06/26/25 18:14:35
============================================================
time = 3.30813E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.3058503E-01 *Ei/E0 = 7.4670687E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.364E+04 exceeds prior maximum 2.030E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.4620559E-02 *Ei/E0 = 2.8520158E-01
Iteration: 3 *|du|/|u| = 4.2282435E-03 *Ei/E0 = 3.1368954E-03
Iteration: 4 *|du|/|u| = 4.0875001E-03 *Ei/E0 = 1.5137001E-03
Iteration: 5 *|du|/|u| = 3.1883083E-03 *Ei/E0 = 9.9629965E-04
Iteration: 6 *|du|/|u| = 6.5158146E-03 *Ei/E0 = 1.4331282E-03
Iteration: 7 *|du|/|u| = 6.8525762E-03 *Ei/E0 = 2.7538699E-03
Iteration: 8 *|du|/|u| = 2.2784434E-03 *Ei/E0 = 1.6359171E-03
Iteration: 9 *|du|/|u| = 3.6010197E-03 *Ei/E0 = 1.3978708E-03
Iteration: 10 *|du|/|u| = 2.1997617E-03 *Ei/E0 = 1.1255202E-03
Iteration: 11 *|du|/|u| = 2.2883148E-03 *Ei/E0 = 9.1701829E-04
Iteration: 12 *|du|/|u| = 2.1084263E-03 *Ei/E0 = 7.0397548E-04
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.3450126E-03 *Ei/E0 = 5.6531785E-04
Iteration: 14 *|du|/|u| = 7.9091744E-04 *Ei/E0 = 1.1748376E-04
Equilibrium established after 14 iterations 06/26/25 18:14:36
3465 t 3.3081E-01 dt 2.15E-05 write d3plot file 06/26/25 18:14:36
BEGIN implicit dynamics step 50 t= 3.3083E-01 06/26/25 18:14:36
============================================================
time = 3.30835E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.2592956E-01 *Ei/E0 = 8.4079072E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.551E+04 exceeds prior maximum 2.153E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.4583027E-02 *Ei/E0 = 3.3810744E-01
Iteration: 3 *|du|/|u| = 4.4709143E-03 *Ei/E0 = 4.4298589E-03
Iteration: 4 *|du|/|u| = 4.1627146E-03 *Ei/E0 = 2.1284389E-03
Iteration: 5 *|du|/|u| = 1.3545053E-03 *Ei/E0 = 4.8691623E-04
Iteration: 6 *|du|/|u| = 1.7868481E-03 *Ei/E0 = 2.8127729E-04
Iteration: 7 *|du|/|u| = 1.8988306E-03 *Ei/E0 = 3.3059095E-04
Iteration: 8 *|du|/|u| = 2.9356827E-03 *Ei/E0 = 4.1402400E-04
Iteration: 9 *|du|/|u| = 1.6918675E-03 *Ei/E0 = 7.3126388E-04
Iteration: 10 *|du|/|u| = 1.3880647E-03 *Ei/E0 = 3.5177924E-04
Iteration: 11 *|du|/|u| = 1.5462782E-03 *Ei/E0 = 2.3511445E-04
Iteration: 12 *|du|/|u| = 1.1892878E-03 *Ei/E0 = 1.6792511E-04
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 5.6160492E-04 *Ei/E0 = 9.4932463E-05
Equilibrium established after 13 iterations 06/26/25 18:14:37
BEGIN implicit dynamics step 51 t= 3.3086E-01 06/26/25 18:14:37
============================================================
time = 3.30856E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 1.1745433E-01 *Ei/E0 = 8.7764010E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.504E+04 exceeds prior maximum 2.255E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.1324273E-02 *Ei/E0 = 2.7361106E-01
Iteration: 3 *|du|/|u| = 3.1649879E-03 *Ei/E0 = 2.7285228E-03
Iteration: 4 *|du|/|u| = 2.5609541E-03 *Ei/E0 = 1.0965298E-03
Iteration: 5 *|du|/|u| = 7.7818934E-04 *Ei/E0 = 1.9397060E-04
Equilibrium established after 5 iterations 06/26/25 18:14:37
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 2.15443E-05 dt(new) = 3.41455E-05
------------------------------------------------------------
BEGIN implicit dynamics step 52 t= 3.3089E-01 06/26/25 18:14:37
============================================================
time = 3.30890E-01
current step size = 3.41455E-05
Iteration: 1 *|du|/|u| = 1.5292005E-01 *Ei/E0 = 7.7271767E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.024E+04 exceeds prior maximum 1.623E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 4.7324726E-02 *Ei/E0 = 2.1059028E-01
Iteration: 3 *|du|/|u| = 1.1886189E-02 *Ei/E0 = 2.5370106E-02
Iteration: 4 *|du|/|u| = 1.1539345E-02 *Ei/E0 = 1.4936988E-02
Iteration: 5 *|du|/|u| = 1.4318575E-02 *Ei/E0 = 2.7950041E-02
Iteration: 6 *|du|/|u| = 1.2148057E-02 *Ei/E0 = 1.6282896E-02
Iteration: 7 *|du|/|u| = 1.3417861E-02 *Ei/E0 = 2.1854656E-02
Iteration: 8 *|du|/|u| = 9.3191123E-03 *Ei/E0 = 7.1400978E-03
Iteration: 9 *|du|/|u| = 1.2065810E-02 *Ei/E0 = 9.6606420E-03
Iteration: 10 *|du|/|u| = 7.5034666E-03 *Ei/E0 = 8.3047170E-03
Iteration: 11 *|du|/|u| = 8.2415470E-03 *Ei/E0 = 5.2615921E-03
Iteration: 12 *|du|/|u| = 6.3376240E-03 *Ei/E0 = 4.1853647E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 4.5163668E-03 *Ei/E0 = 3.1095492E-03
Iteration: 14 *|du|/|u| = 4.7845116E-03 *Ei/E0 = 1.7753837E-03
Iteration: 15 *|du|/|u| = 2.3456689E-03 *Ei/E0 = 1.3952888E-03
Iteration: 16 *|du|/|u| = 2.0974674E-03 *Ei/E0 = 7.3888328E-04
Iteration: 17 *|du|/|u| = 3.2274825E-03 *Ei/E0 = 5.7739598E-04
Iteration: 18 *|du|/|u| = 2.2954359E-03 *Ei/E0 = 3.9840611E-04
Iteration: 19 *|du|/|u| = 1.2337209E-03 *Ei/E0 = 3.1406592E-04
Iteration: 20 *|du|/|u| = 1.2053686E-03 *Ei/E0 = 3.4330385E-04
Iteration: 21 *|du|/|u| = 8.6957265E-04 *Ei/E0 = 2.0256680E-04
Equilibrium established after 21 iterations 06/26/25 18:14:39
------------------------------------------------------------
Converged in more than ITEOPT+ITEWIN= 16 iterations,
automatic time step size DECREASE:
dt(old) = 3.41455E-05 dt(new) = 1.71133E-05
------------------------------------------------------------
BEGIN implicit dynamics step 53 t= 3.3091E-01 06/26/25 18:14:39
============================================================
time = 3.30908E-01
current step size = 1.71133E-05
Iteration: 1 *|du|/|u| = 8.4036237E-02 *Ei/E0 = 1.0000000E+00
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.136E+04 exceeds prior maximum 3.114E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 7.9442944E-03 *Ei/E0 = 1.6550187E-01
Iteration: 3 *|du|/|u| = 2.2573237E-03 *Ei/E0 = 2.9083356E-03
Iteration: 4 *|du|/|u| = 1.7540213E-03 *Ei/E0 = 1.0216533E-03
Iteration: 5 *|du|/|u| = 4.1228560E-04 *Ei/E0 = 1.4137149E-04
Equilibrium established after 5 iterations 06/26/25 18:14:39
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.71133E-05 dt(new) = 2.71227E-05
------------------------------------------------------------
3563 t 3.3091E-01 dt 1.71E-05 write d3plot file 06/26/25 18:14:39
BEGIN implicit dynamics step 54 t= 3.3093E-01 06/26/25 18:14:39
============================================================
time = 3.30935E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 1.1735086E-01 *Ei/E0 = 9.9842300E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.098E+04 exceeds prior maximum 2.284E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.2743785E-02 *Ei/E0 = 5.7683198E-01
Iteration: 3 *|du|/|u| = 4.6597800E-03 *Ei/E0 = 1.0103630E-02
Iteration: 4 *|du|/|u| = 4.8633909E-03 *Ei/E0 = 5.7851905E-03
Iteration: 5 *|du|/|u| = 4.3920743E-03 *Ei/E0 = 3.7988077E-03
Iteration: 6 *|du|/|u| = 3.4485422E-03 *Ei/E0 = 4.3847490E-03
Iteration: 7 *|du|/|u| = 4.1174030E-03 *Ei/E0 = 2.5280243E-03
Iteration: 8 *|du|/|u| = 2.0020519E-03 *Ei/E0 = 3.4948803E-03
Iteration: 9 *|du|/|u| = 2.3693776E-03 *Ei/E0 = 2.5914653E-03
Iteration: 10 *|du|/|u| = 3.0356579E-03 *Ei/E0 = 2.0906424E-03
Iteration: 11 *|du|/|u| = 3.1853453E-03 *Ei/E0 = 3.2737849E-03
Iteration: 12 *|du|/|u| = 2.1990244E-03 *Ei/E0 = 1.8559481E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 2.3980707E-03 *Ei/E0 = 1.9581791E-03
Iteration: 14 *|du|/|u| = 2.9532035E-03 *Ei/E0 = 1.0172910E-03
Iteration: 15 *|du|/|u| = 9.9852969E-04 *Ei/E0 = 7.1592509E-04
Equilibrium established after 15 iterations 06/26/25 18:14:40
BEGIN implicit dynamics step 55 t= 3.3096E-01 06/26/25 18:14:40
============================================================
time = 3.30962E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 1.2001773E-01 *Ei/E0 = 8.3343824E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.095E+04 exceeds prior maximum 2.308E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 4.1507555E-02 *Ei/E0 = 2.5342168E-01
Iteration: 3 *|du|/|u| = 8.0586423E-03 *Ei/E0 = 2.5799868E-02
Iteration: 4 *|du|/|u| = 5.2035258E-03 *Ei/E0 = 1.1200353E-02
Iteration: 5 *|du|/|u| = 7.9755772E-03 *Ei/E0 = 1.0306334E-02
Iteration: 6 *|du|/|u| = 2.6153918E-03 *Ei/E0 = 1.7282477E-03
Iteration: 7 *|du|/|u| = 4.1613936E-03 *Ei/E0 = 3.7203535E-03
Iteration: 8 *|du|/|u| = 2.6004086E-03 *Ei/E0 = 2.6879471E-03
Iteration: 9 *|du|/|u| = 3.0304409E-03 *Ei/E0 = 1.9342300E-03
Iteration: 10 *|du|/|u| = 1.6222729E-03 *Ei/E0 = 8.3049172E-04
Iteration: 11 *|du|/|u| = 1.3922067E-03 *Ei/E0 = 8.3311885E-04
Iteration: 12 *|du|/|u| = 1.9478192E-03 *Ei/E0 = 9.4412065E-04
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.0620079E-03 *Ei/E0 = 5.4170662E-04
Iteration: 14 *|du|/|u| = 9.9883620E-04 *Ei/E0 = 1.6624395E-04
Equilibrium established after 14 iterations 06/26/25 18:14:42
BEGIN implicit dynamics step 56 t= 3.3099E-01 06/26/25 18:14:42
============================================================
time = 3.30989E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 1.0653237E-01 *Ei/E0 = 8.3957406E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.029E+04 exceeds prior maximum 2.422E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 9.7307329E-03 *Ei/E0 = 3.8182065E-01
Iteration: 3 *|du|/|u| = 2.7604650E-03 *Ei/E0 = 4.6436878E-03
Iteration: 4 *|du|/|u| = 3.5446704E-03 *Ei/E0 = 2.1273606E-03
Iteration: 5 *|du|/|u| = 2.7109384E-03 *Ei/E0 = 2.1134662E-03
Iteration: 6 *|du|/|u| = 1.2505781E-03 *Ei/E0 = 1.0291801E-03
Iteration: 7 *|du|/|u| = 1.0785587E-03 *Ei/E0 = 5.1801580E-04
Iteration: 8 *|du|/|u| = 1.3109697E-03 *Ei/E0 = 5.0136349E-04
Iteration: 9 *|du|/|u| = 1.7842139E-03 *Ei/E0 = 5.2316025E-04
Iteration: 10 *|du|/|u| = 6.1840728E-04 *Ei/E0 = 3.3482600E-04
Equilibrium established after 10 iterations 06/26/25 18:14:42
BEGIN implicit dynamics step 57 t= 3.3102E-01 06/26/25 18:14:42
============================================================
time = 3.31016E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 9.5875524E-02 *Ei/E0 = 7.8400678E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.739E+04 exceeds prior maximum 2.387E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 9.3359367E-03 *Ei/E0 = 2.8258299E-01
Iteration: 3 *|du|/|u| = 3.0926883E-03 *Ei/E0 = 5.4174052E-03
Iteration: 4 *|du|/|u| = 3.3703116E-03 *Ei/E0 = 2.5807371E-03
Iteration: 5 *|du|/|u| = 1.4347663E-03 *Ei/E0 = 1.6256720E-03
Iteration: 6 *|du|/|u| = 1.6618551E-03 *Ei/E0 = 1.5616594E-03
Iteration: 7 *|du|/|u| = 1.0983851E-03 *Ei/E0 = 7.1784843E-04
Iteration: 8 *|du|/|u| = 7.2593065E-04 *Ei/E0 = 3.0817972E-04
Equilibrium established after 8 iterations 06/26/25 18:14:43
3652 t 3.3102E-01 dt 2.71E-05 write d3plot file 06/26/25 18:14:43
BEGIN implicit dynamics step 58 t= 3.3104E-01 06/26/25 18:14:43
============================================================
time = 3.31043E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 8.8561952E-02 *Ei/E0 = 7.8935845E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.526E+04 exceeds prior maximum 2.386E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 8.6732501E-03 *Ei/E0 = 2.3949863E-01
Iteration: 3 *|du|/|u| = 5.4028854E-03 *Ei/E0 = 1.4820484E-02
Iteration: 4 *|du|/|u| = 3.5605899E-03 *Ei/E0 = 8.0652912E-03
Iteration: 5 *|du|/|u| = 4.2891758E-03 *Ei/E0 = 3.0876534E-03
Iteration: 6 *|du|/|u| = 1.1704003E-03 *Ei/E0 = 1.1785344E-03
Iteration: 7 *|du|/|u| = 2.6717235E-03 *Ei/E0 = 1.9254904E-03
Iteration: 8 *|du|/|u| = 1.1726060E-03 *Ei/E0 = 1.7694110E-03
Iteration: 9 *|du|/|u| = 9.3174951E-04 *Ei/E0 = 6.4764872E-04
Equilibrium established after 9 iterations 06/26/25 18:14:43
BEGIN implicit dynamics step 59 t= 3.3107E-01 06/26/25 18:14:43
============================================================
time = 3.31070E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 8.0579140E-02 *Ei/E0 = 7.6598127E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.636E+04 exceeds prior maximum 2.394E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 8.1162307E-03 *Ei/E0 = 3.1739620E-01
Iteration: 3 *|du|/|u| = 4.6024918E-03 *Ei/E0 = 1.3365028E-02
Iteration: 4 *|du|/|u| = 3.9752283E-03 *Ei/E0 = 7.6573859E-03
Iteration: 5 *|du|/|u| = 3.0704624E-03 *Ei/E0 = 9.5835142E-03
Iteration: 6 *|du|/|u| = 1.4874062E-03 *Ei/E0 = 2.4048115E-03
Iteration: 7 *|du|/|u| = 2.5905300E-03 *Ei/E0 = 2.3018796E-03
Iteration: 8 *|du|/|u| = 4.0602853E-03 *Ei/E0 = 2.2307620E-03
Iteration: 9 *|du|/|u| = 1.5460413E-03 *Ei/E0 = 1.4575636E-03
Iteration: 10 *|du|/|u| = 1.3940090E-03 *Ei/E0 = 1.4442707E-03
Iteration: 11 *|du|/|u| = 1.1789705E-03 *Ei/E0 = 1.0596582E-03
Iteration: 12 *|du|/|u| = 8.6647444E-04 *Ei/E0 = 9.6109360E-04
Equilibrium established after 12 iterations 06/26/25 18:14:44
BEGIN implicit dynamics step 60 t= 3.3110E-01 06/26/25 18:14:44
============================================================
time = 3.31097E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 7.8143627E-02 *Ei/E0 = 7.9076643E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.839E+03 exceeds prior maximum 2.446E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.6553001E-02 *Ei/E0 = 2.3757558E-01
Iteration: 3 *|du|/|u| = 6.5557235E-03 *Ei/E0 = 3.1949834E-02
Iteration: 4 *|du|/|u| = 3.3348313E-03 *Ei/E0 = 1.4004995E-02
Iteration: 5 *|du|/|u| = 8.6735802E-03 *Ei/E0 = 1.7029817E-02
Iteration: 6 *|du|/|u| = 1.9049131E-03 *Ei/E0 = 1.1735218E-03
Iteration: 7 *|du|/|u| = 1.9235833E-03 *Ei/E0 = 3.2745415E-03
Iteration: 8 *|du|/|u| = 2.6585794E-03 *Ei/E0 = 2.6945163E-03
Iteration: 9 *|du|/|u| = 1.4001628E-03 *Ei/E0 = 1.3620945E-03
Iteration: 10 *|du|/|u| = 1.8882625E-03 *Ei/E0 = 1.1672251E-03
Iteration: 11 *|du|/|u| = 1.2469595E-03 *Ei/E0 = 7.1278576E-04
Iteration: 12 *|du|/|u| = 1.2164548E-03 *Ei/E0 = 9.0574592E-04
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.3742463E-03 *Ei/E0 = 8.8681099E-04
Iteration: 14 *|du|/|u| = 4.8275794E-02 *Ei/E0 = 3.4396444E-02
Iteration: 15 *|du|/|u| = 2.2113898E-03 *Ei/E0 = 2.1848173E-03
Iteration: 16 *|du|/|u| = 9.7126545E-04 *Ei/E0 = 2.5809308E-03
Equilibrium established after 16 iterations 06/26/25 18:14:46
BEGIN implicit dynamics step 61 t= 3.3112E-01 06/26/25 18:14:46
============================================================
time = 3.31124E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 7.3462582E-02 *Ei/E0 = 8.0406387E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.740E+03 exceeds prior maximum 2.846E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.3990577E-02 *Ei/E0 = 2.2176770E-01
Iteration: 3 *|du|/|u| = 3.7828754E-03 *Ei/E0 = 1.5784745E-02
Iteration: 4 *|du|/|u| = 3.6681087E-03 *Ei/E0 = 7.8444257E-03
Iteration: 5 *|du|/|u| = 2.4427075E-03 *Ei/E0 = 3.7275328E-03
Iteration: 6 *|du|/|u| = 1.4825050E-03 *Ei/E0 = 1.6783180E-03
Iteration: 7 *|du|/|u| = 1.5258044E-03 *Ei/E0 = 1.5821966E-03
Iteration: 8 *|du|/|u| = 1.1664525E-03 *Ei/E0 = 1.3936840E-03
Iteration: 9 *|du|/|u| = 9.5010170E-04 *Ei/E0 = 1.1445568E-03
Equilibrium established after 9 iterations 06/26/25 18:14:46
3753 t 3.3112E-01 dt 2.71E-05 write d3plot file 06/26/25 18:14:46
BEGIN implicit dynamics step 62 t= 3.3115E-01 06/26/25 18:14:46
============================================================
time = 3.31152E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 6.8182123E-02 *Ei/E0 = 7.9211841E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.457E+04 exceeds prior maximum 2.553E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 6.7093129E-03 *Ei/E0 = 3.5759413E-01
Iteration: 3 *|du|/|u| = 3.4953467E-03 *Ei/E0 = 1.6578118E-02
Iteration: 4 *|du|/|u| = 2.8357972E-03 *Ei/E0 = 9.5709175E-03
Iteration: 5 *|du|/|u| = 2.3444167E-03 *Ei/E0 = 5.5374484E-03
Iteration: 6 *|du|/|u| = 1.7196128E-03 *Ei/E0 = 4.6226557E-03
Iteration: 7 *|du|/|u| = 1.2545123E-03 *Ei/E0 = 1.8904291E-03
Iteration: 8 *|du|/|u| = 2.3531455E-03 *Ei/E0 = 1.8016338E-03
Iteration: 9 *|du|/|u| = 1.7742806E-03 *Ei/E0 = 3.4185483E-03
Iteration: 10 *|du|/|u| = 1.7472378E-03 *Ei/E0 = 2.2359608E-03
Iteration: 11 *|du|/|u| = 1.0636316E-03 *Ei/E0 = 1.1058002E-03
Iteration: 12 *|du|/|u| = 7.5148234E-04 *Ei/E0 = 8.9717635E-04
Equilibrium established after 12 iterations 06/26/25 18:14:47
BEGIN implicit dynamics step 63 t= 3.3118E-01 06/26/25 18:14:47
============================================================
time = 3.31179E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 6.7834231E-02 *Ei/E0 = 8.5779189E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.606E+03 exceeds prior maximum 2.608E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.2168921E-02 *Ei/E0 = 2.4190137E-01
Iteration: 3 *|du|/|u| = 5.4136534E-03 *Ei/E0 = 3.4209330E-02
Iteration: 4 *|du|/|u| = 3.1115666E-03 *Ei/E0 = 2.0256457E-02
Iteration: 5 *|du|/|u| = 5.7640634E-03 *Ei/E0 = 1.7955908E-02
Iteration: 6 *|du|/|u| = 1.5809739E-03 *Ei/E0 = 2.2019296E-03
Iteration: 7 *|du|/|u| = 2.6641061E-03 *Ei/E0 = 5.4996757E-03
Iteration: 8 *|du|/|u| = 1.7771455E-03 *Ei/E0 = 5.3372389E-03
Iteration: 9 *|du|/|u| = 2.5390273E-03 *Ei/E0 = 3.4277926E-03
Iteration: 10 *|du|/|u| = 1.1494703E-03 *Ei/E0 = 1.5577112E-03
Iteration: 11 *|du|/|u| = 1.7182967E-03 *Ei/E0 = 2.1845625E-03
Iteration: 12 *|du|/|u| = 1.8783159E-03 *Ei/E0 = 1.7421712E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 9.2416471E-04 *Ei/E0 = 2.0989389E-03
Equilibrium established after 13 iterations 06/26/25 18:14:48
BEGIN implicit dynamics step 64 t= 3.3121E-01 06/26/25 18:14:48
============================================================
time = 3.31206E-01
current step size = 2.71227E-05
Iteration: 1 *|du|/|u| = 6.4474954E-02 *Ei/E0 = 8.8554422E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.447E+04 exceeds prior maximum 2.445E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 5.2193604E-03 *Ei/E0 = 3.9434154E-01
Iteration: 3 *|du|/|u| = 2.0713747E-03 *Ei/E0 = 6.9382532E-03
Iteration: 4 *|du|/|u| = 2.1605362E-03 *Ei/E0 = 3.3228988E-03
Iteration: 5 *|du|/|u| = 8.6875402E-04 *Ei/E0 = 1.8475736E-03
Equilibrium established after 5 iterations 06/26/25 18:14:49
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 2.71227E-05 dt(new) = 4.29866E-05
------------------------------------------------------------
3817 t 3.3121E-01 dt 2.71E-05 write d3plot file 06/26/25 18:14:49
BEGIN implicit dynamics step 65 t= 3.3125E-01 06/26/25 18:14:49
============================================================
time = 3.31249E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 9.1153567E-02 *Ei/E0 = 8.4919339E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.810E+04 exceeds prior maximum 1.868E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 7.1691431E-03 *Ei/E0 = 3.3094137E-01
Iteration: 3 *|du|/|u| = 3.6439148E-03 *Ei/E0 = 1.6484448E-02
Iteration: 4 *|du|/|u| = 4.6833003E-03 *Ei/E0 = 8.6017306E-03
Iteration: 5 *|du|/|u| = 5.4263558E-03 *Ei/E0 = 1.7263487E-02
Iteration: 6 *|du|/|u| = 3.6712370E-03 *Ei/E0 = 1.0614905E-02
Iteration: 7 *|du|/|u| = 3.1442026E-03 *Ei/E0 = 8.0917052E-03
Iteration: 8 *|du|/|u| = 4.0989955E-03 *Ei/E0 = 7.5365648E-03
Iteration: 9 *|du|/|u| = 4.5278148E-03 *Ei/E0 = 7.9504020E-03
Iteration: 10 *|du|/|u| = 4.0051163E-03 *Ei/E0 = 7.6847846E-03
Iteration: 11 *|du|/|u| = 2.5794170E-03 *Ei/E0 = 4.8215271E-03
Iteration: 12 *|du|/|u| = 3.0230578E-03 *Ei/E0 = 4.2422658E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 2.6500806E-03 *Ei/E0 = 2.7787120E-03
Iteration: 14 *|du|/|u| = 2.7948562E-03 *Ei/E0 = 2.9023447E-03
Iteration: 15 *|du|/|u| = 9.7819512E-04 *Ei/E0 = 3.0362904E-03
Equilibrium established after 15 iterations 06/26/25 18:14:50
BEGIN implicit dynamics step 66 t= 3.3129E-01 06/26/25 18:14:50
============================================================
time = 3.31292E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 9.2227580E-02 *Ei/E0 = 5.1697419E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.786E+03 exceeds prior maximum 1.919E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 3.8129418E-02 *Ei/E0 = 1.9158826E-01
Iteration: 3 *|du|/|u| = 1.3801899E-02 *Ei/E0 = 6.1657398E-02
Iteration: 4 *|du|/|u| = 2.7042416E-03 *Ei/E0 = 7.9028727E-03
Iteration: 5 *|du|/|u| = 5.6970845E-03 *Ei/E0 = 8.4689838E-03
Iteration: 6 *|du|/|u| = 5.4742615E-03 *Ei/E0 = 9.3630914E-03
Iteration: 7 *|du|/|u| = 3.0580500E-03 *Ei/E0 = 6.1154680E-03
Iteration: 8 *|du|/|u| = 3.0100487E-03 *Ei/E0 = 4.0997616E-03
Iteration: 9 *|du|/|u| = 4.1581440E-03 *Ei/E0 = 5.3166262E-03
Iteration: 10 *|du|/|u| = 2.3700611E-03 *Ei/E0 = 1.9537327E-03
Iteration: 11 *|du|/|u| = 2.1707068E-03 *Ei/E0 = 1.8780309E-03
Iteration: 12 *|du|/|u| = 2.0664526E-03 *Ei/E0 = 1.5916634E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 2.0977081E-03 *Ei/E0 = 1.4926542E-03
Iteration: 14 *|du|/|u| = 2.1705039E-03 *Ei/E0 = 1.0284620E-03
Iteration: 15 *|du|/|u| = 1.0365651E-03 *Ei/E0 = 7.5616720E-04
Iteration: 16 *|du|/|u| = 7.9471883E-04 *Ei/E0 = 5.1679324E-04
Equilibrium established after 16 iterations 06/26/25 18:14:52
BEGIN implicit dynamics step 67 t= 3.3133E-01 06/26/25 18:14:52
============================================================
time = 3.31335E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 8.4189725E-02 *Ei/E0 = 5.2539785E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.626E+04 exceeds prior maximum 1.811E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 5.3024088E-03 *Ei/E0 = 1.4177396E-01
Iteration: 3 *|du|/|u| = 3.2015899E-03 *Ei/E0 = 5.3121991E-03
Iteration: 4 *|du|/|u| = 2.8296151E-03 *Ei/E0 = 3.5270554E-03
Iteration: 5 *|du|/|u| = 3.4807210E-03 *Ei/E0 = 3.9774919E-03
Iteration: 6 *|du|/|u| = 3.0677997E-03 *Ei/E0 = 3.2499806E-03
Iteration: 7 *|du|/|u| = 2.9276733E-03 *Ei/E0 = 3.0508569E-03
Iteration: 8 *|du|/|u| = 2.4374471E-03 *Ei/E0 = 6.6116237E-03
Iteration: 9 *|du|/|u| = 3.8424593E-03 *Ei/E0 = 4.9864329E-03
Iteration: 10 *|du|/|u| = 3.4646475E-03 *Ei/E0 = 6.6191320E-03
Iteration: 11 *|du|/|u| = 3.0188912E-03 *Ei/E0 = 3.6302453E-03
Iteration: 12 *|du|/|u| = 2.5996644E-03 *Ei/E0 = 7.3012575E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 3.1645626E-03 *Ei/E0 = 7.1270315E-03
Iteration: 14 *|du|/|u| = 1.5348278E-03 *Ei/E0 = 2.2990166E-03
Iteration: 15 *|du|/|u| = 9.0565046E-04 *Ei/E0 = 1.1224007E-03
Equilibrium established after 15 iterations 06/26/25 18:14:53
3961 t 3.3133E-01 dt 4.30E-05 write d3plot file 06/26/25 18:14:53
BEGIN implicit dynamics step 68 t= 3.3138E-01 06/26/25 18:14:53
============================================================
time = 3.31378E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 8.1331599E-02 *Ei/E0 = 5.8345008E-01
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.904E+04 exceeds prior maximum 1.931E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 6.8344655E-03 *Ei/E0 = 2.1210908E-01
Iteration: 3 *|du|/|u| = 1.2940933E-02 *Ei/E0 = 8.3460038E-02
Iteration: 4 *|du|/|u| = 2.6630497E-03 *Ei/E0 = 6.7136821E-03
Iteration: 5 *|du|/|u| = 3.9571790E-03 *Ei/E0 = 6.0250177E-03
Iteration: 6 *|du|/|u| = 4.4365526E-03 *Ei/E0 = 8.9931850E-03
Iteration: 7 *|du|/|u| = 2.8366311E-03 *Ei/E0 = 5.7814666E-03
Iteration: 8 *|du|/|u| = 2.8267817E-03 *Ei/E0 = 5.3618504E-03
Iteration: 9 *|du|/|u| = 2.3398737E-03 *Ei/E0 = 4.0556859E-03
Iteration: 10 *|du|/|u| = 2.8316289E-03 *Ei/E0 = 3.3200269E-03
Iteration: 11 *|du|/|u| = 1.8461641E-03 *Ei/E0 = 3.4743167E-03
Iteration: 12 *|du|/|u| = 3.3151178E-03 *Ei/E0 = 3.3155147E-03
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 1.9418903E-03 *Ei/E0 = 3.2892121E-03
Iteration: 14 *|du|/|u| = 1.3658862E-03 *Ei/E0 = 1.6550104E-03
Iteration: 15 *|du|/|u| = 9.0224836E-04 *Ei/E0 = 6.4544564E-04
Equilibrium established after 15 iterations 06/26/25 18:14:55
BEGIN implicit dynamics step 69 t= 3.3142E-01 06/26/25 18:14:55
============================================================
time = 3.31421E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 7.8887414E-02 *Ei/E0 = 4.2046772E-02
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.702E+03 exceeds prior maximum 1.898E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 3.4695289E-02 *Ei/E0 = 1.0200558E-02
Iteration: 3 *|du|/|u| = 4.3706451E-03 *Ei/E0 = 9.9720662E-04
Iteration: 4 *|du|/|u| = 3.0805532E-03 *Ei/E0 = 4.8646195E-04
Iteration: 5 *|du|/|u| = 3.6226357E-03 *Ei/E0 = 3.9808396E-04
Iteration: 6 *|du|/|u| = 2.2082573E-03 *Ei/E0 = 2.1324054E-04
Iteration: 7 *|du|/|u| = 2.9044867E-03 *Ei/E0 = 4.3910142E-04
Iteration: 8 *|du|/|u| = 2.9821569E-03 *Ei/E0 = 4.9099872E-04
Iteration: 9 *|du|/|u| = 4.7079734E-03 *Ei/E0 = 5.6518308E-04
Iteration: 10 *|du|/|u| = 2.0584978E-03 *Ei/E0 = 2.2908563E-04
Iteration: 11 *|du|/|u| = 1.4748524E-03 *Ei/E0 = 1.5932101E-04
Iteration: 12 *|du|/|u| = 1.6756174E-03 *Ei/E0 = 2.2625350E-04
ITERATION LIMIT reached, automatically REFORMING stiffness matrix...
Iteration: 13 *|du|/|u| = 2.1135753E-03 *Ei/E0 = 2.8469647E-04
Iteration: 14 *|du|/|u| = 5.9014904E-03 *Ei/E0 = 6.7984690E-04
Iteration: 15 *|du|/|u| = 7.8429733E-04 *Ei/E0 = 5.5008257E-05
Equilibrium established after 15 iterations 06/26/25 18:14:56
4061 t 3.3142E-01 dt 4.30E-05 write d3plot file 06/26/25 18:14:56
BEGIN implicit dynamics step 70 t= 3.3146E-01 06/26/25 18:14:56
============================================================
time = 3.31464E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 7.3613552E-02 *Ei/E0 = 8.8687537E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.553E+03 exceeds prior maximum 2.065E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 5.9319353E-02 *Ei/E0 = 6.8535643E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.748E+03 exceeds prior maximum 7.553E+03
automatically REFORMING stiffness matrix...
Iteration: 3 *|du|/|u| = 3.9288588E-03 *Ei/E0 = 8.0240665E-04
Iteration: 4 *|du|/|u| = 5.0575195E-03 *Ei/E0 = 2.9656186E-04
Iteration: 5 *|du|/|u| = 1.6092982E-03 *Ei/E0 = 4.0037039E-05
Iteration: 6 *|du|/|u| = 1.7015545E-03 *Ei/E0 = 3.2138728E-05
Iteration: 7 *|du|/|u| = 1.2557873E-03 *Ei/E0 = 2.1986221E-05
Iteration: 8 *|du|/|u| = 9.8822790E-04 *Ei/E0 = 1.4982727E-05
Equilibrium established after 8 iterations 06/26/25 18:14:57
BEGIN implicit dynamics step 71 t= 3.3151E-01 06/26/25 18:14:57
============================================================
time = 3.31507E-01
current step size = 4.29866E-05
Iteration: 1 *|du|/|u| = 6.6908353E-02 *Ei/E0 = 8.4132253E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.078E+03 exceeds prior maximum 1.839E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 2005 vs 2062
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 4.29866E-05 dt(new) = 1.99526E-05
------------------------------------------------------------
BEGIN implicit dynamics step 71 t= 3.3148E-01 06/26/25 18:14:58
============================================================
time = 3.31484E-01
current step size = 1.99526E-05
Iteration: 1 *|du|/|u| = 3.2225968E-02 *Ei/E0 = 8.8737209E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 5.945E+03 exceeds prior maximum 3.382E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 2005 vs 2062
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.99526E-05 dt(new) = 1.00000E-05
------------------------------------------------------------
BEGIN implicit dynamics step 71 t= 3.3147E-01 06/26/25 18:14:58
============================================================
time = 3.31474E-01
current step size = 1.00000E-05
Iteration: 1 *|du|/|u| = 1.6750110E-02 *Ei/E0 = 9.1057684E-03
Iteration: 2 *|du|/|u| = 9.3823669E-04 *Ei/E0 = 1.1776807E-04
Equilibrium established after 2 iterations 06/26/25 18:14:58
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.00000E-05 dt(new) = 1.58489E-05
------------------------------------------------------------
BEGIN implicit dynamics step 72 t= 3.3149E-01 06/26/25 18:14:58
============================================================
time = 3.31490E-01
current step size = 1.58489E-05
Iteration: 1 *|du|/|u| = 2.5776606E-02 *Ei/E0 = 9.0600180E-03
Iteration: 2 *|du|/|u| = 1.1610351E-03 *Ei/E0 = 1.1599222E-04
Iteration: 3 *|du|/|u| = 8.3959700E-04 *Ei/E0 = 3.2947839E-05
Equilibrium established after 3 iterations 06/26/25 18:14:59
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.58489E-05 dt(new) = 2.51189E-05
------------------------------------------------------------
BEGIN implicit dynamics step 73 t= 3.3151E-01 06/26/25 18:14:59
============================================================
time = 3.31515E-01
current step size = 2.51189E-05
Iteration: 1 *|du|/|u| = 3.8694831E-02 *Ei/E0 = 8.8168080E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.576E+04 exceeds prior maximum 2.704E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.3722661E-03 *Ei/E0 = 1.1288799E-03
Iteration: 3 *|du|/|u| = 1.5420285E-03 *Ei/E0 = 2.6690572E-04
Iteration: 4 *|du|/|u| = 9.9913245E-04 *Ei/E0 = 5.0702416E-05
Equilibrium established after 4 iterations 06/26/25 18:14:59
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 2.51189E-05 dt(new) = 3.98107E-05
------------------------------------------------------------
4111 t 3.3151E-01 dt 2.51E-05 write d3plot file 06/26/25 18:14:59
BEGIN implicit dynamics step 74 t= 3.3155E-01 06/26/25 18:14:59
============================================================
time = 3.31555E-01
current step size = 3.98107E-05
Iteration: 1 *|du|/|u| = 5.5095033E-02 *Ei/E0 = 8.2327885E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.104E+04 exceeds prior maximum 2.075E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 13 vs 1995
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 3.98107E-05 dt(new) = 1.84785E-05
------------------------------------------------------------
BEGIN implicit dynamics step 74 t= 3.3153E-01 06/26/25 18:14:59
============================================================
time = 3.31533E-01
current step size = 1.84785E-05
Iteration: 1 *|du|/|u| = 2.7603456E-02 *Ei/E0 = 8.7156809E-03
Iteration: 2 *|du|/|u| = 1.7019462E-03 *Ei/E0 = 1.9141181E-04
Iteration: 3 *|du|/|u| = 9.2915377E-04 *Ei/E0 = 1.4474130E-04
Equilibrium established after 3 iterations 06/26/25 18:15:00
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.84785E-05 dt(new) = 2.92864E-05
------------------------------------------------------------
BEGIN implicit dynamics step 75 t= 3.3156E-01 06/26/25 18:15:00
============================================================
time = 3.31563E-01
current step size = 2.92864E-05
Iteration: 1 *|du|/|u| = 4.2146464E-02 *Ei/E0 = 8.4146681E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.930E+03 exceeds prior maximum 3.232E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.9331028E-02 *Ei/E0 = 4.4457107E-03
Iteration: 3 *|du|/|u| = 1.8362047E+00 *Ei/E0 = 2.9276596E-01
Iteration: 4 *|du|/|u| = 1.5729909E-03 *Ei/E0 = 1.3481793E-04
Iteration: 5 *|du|/|u| = 1.5766149E-03 *Ei/E0 = 6.6527846E-05
Iteration: 6 *|du|/|u| = 2.0168180E-03 *Ei/E0 = 7.8470986E-05
Iteration: 7 *|du|/|u| = 1.0588730E-03 *Ei/E0 = 3.7687611E-05
Iteration: 8 *|du|/|u| = 1.3532385E-03 *Ei/E0 = 2.5150721E-05
Iteration: 9 *|du|/|u| = 1.4349621E-03 *Ei/E0 = 5.2246558E-05
Iteration: 10 *|du|/|u| = 1.3038207E-03 *Ei/E0 = 3.5352519E-05
Iteration: 11 *|du|/|u| = 8.8585264E-04 *Ei/E0 = 4.0417248E-05
Equilibrium established after 11 iterations 06/26/25 18:15:01
BEGIN implicit dynamics step 76 t= 3.3159E-01 06/26/25 18:15:01
============================================================
time = 3.31592E-01
current step size = 2.92864E-05
Iteration: 1 *|du|/|u| = 3.9656021E-02 *Ei/E0 = 8.4863712E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.189E+04 exceeds prior maximum 3.107E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 1.2807967E-02 *Ei/E0 = 1.4968782E-03
Iteration: 3 *|du|/|u| = 2.4875831E-03 *Ei/E0 = 2.2169107E-04
Iteration: 4 *|du|/|u| = 1.6120017E-03 *Ei/E0 = 1.0669781E-04
Iteration: 5 *|du|/|u| = 1.2895681E-03 *Ei/E0 = 5.8048920E-05
Iteration: 6 *|du|/|u| = 8.1455645E-04 *Ei/E0 = 1.9685534E-05
Equilibrium established after 6 iterations 06/26/25 18:15:02
BEGIN implicit dynamics step 77 t= 3.3162E-01 06/26/25 18:15:02
============================================================
time = 3.31621E-01
current step size = 2.92864E-05
Iteration: 1 *|du|/|u| = 3.6443261E-02 *Ei/E0 = 7.8109082E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 8.222E+03 exceeds prior maximum 3.089E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 3.2623714E-03 *Ei/E0 = 1.3411297E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.067E+03 exceeds prior maximum 8.222E+03
automatically REFORMING stiffness matrix...
Iteration: 3 *|du|/|u| = 2.8232545E-03 *Ei/E0 = 1.1125811E-03
Iteration: 4 *|du|/|u| = 1.8935049E-03 *Ei/E0 = 2.0896004E-04
Iteration: 5 *|du|/|u| = 1.3444926E-03 *Ei/E0 = 1.1643750E-04
Iteration: 6 *|du|/|u| = 3.8444279E-03 *Ei/E0 = 2.3434503E-04
Iteration: 7 *|du|/|u| = 1.4025073E-03 *Ei/E0 = 7.1606665E-05
Iteration: 8 *|du|/|u| = 2.1802039E-03 *Ei/E0 = 1.0795043E-04
Iteration: 9 *|du|/|u| = 2.7484381E-03 *Ei/E0 = 2.1319334E-04
Iteration: 10 *|du|/|u| = 1.8815060E-03 *Ei/E0 = 7.7532801E-05
Iteration: 11 *|du|/|u| = 1.4800274E-03 *Ei/E0 = 8.4362905E-05
Iteration: 12 *|du|/|u| = 8.1867931E-04 *Ei/E0 = 3.0188459E-05
Equilibrium established after 12 iterations 06/26/25 18:15:03
4222 t 3.3162E-01 dt 2.93E-05 write d3plot file 06/26/25 18:15:03
BEGIN implicit dynamics step 78 t= 3.3165E-01 06/26/25 18:15:03
============================================================
time = 3.31650E-01
current step size = 2.92864E-05
Iteration: 1 *|du|/|u| = 3.5691112E-02 *Ei/E0 = 7.8121647E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.345E+04 exceeds prior maximum 2.680E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.6802097E-02 *Ei/E0 = 5.2552302E-03
Iteration: 3 *|du|/|u| = 1.5264159E-02 *Ei/E0 = 2.5550777E-03
Iteration: 4 *|du|/|u| = 9.9742659E-03 *Ei/E0 = 4.7802648E-03
Iteration: 5 *|du|/|u| = 2.5367049E-02 *Ei/E0 = 5.1600265E-03
Iteration: 6 *|du|/|u| = 2.2411739E-02 *Ei/E0 = 3.2921914E-03
Iteration: 7 *|du|/|u| = 5.3932549E-03 *Ei/E0 = 6.8337310E-04
Iteration: 8 *|du|/|u| = 5.1847859E-03 *Ei/E0 = 6.4916731E-04
Iteration: 9 *|du|/|u| = 5.1475717E-03 *Ei/E0 = 2.9758564E-04
Iteration: 10 *|du|/|u| = 4.3852914E-03 *Ei/E0 = 1.6450894E-04
Iteration: 11 *|du|/|u| = 1.5212819E-03 *Ei/E0 = 5.3539702E-05
Iteration: 12 *|du|/|u| = 8.6160757E-04 *Ei/E0 = 5.8172652E-05
Equilibrium established after 12 iterations 06/26/25 18:15:04
BEGIN implicit dynamics step 79 t= 3.3168E-01 06/26/25 18:15:04
============================================================
time = 3.31680E-01
current step size = 2.92864E-05
Iteration: 1 *|du|/|u| = 3.3583674E-02 *Ei/E0 = 7.5297927E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.283E+03 exceeds prior maximum 3.520E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 1995 vs 2033
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 2.92864E-05 dt(new) = 1.35936E-05
------------------------------------------------------------
BEGIN implicit dynamics step 79 t= 3.3166E-01 06/26/25 18:15:05
============================================================
time = 3.31664E-01
current step size = 1.35936E-05
Iteration: 1 *|du|/|u| = 1.6575237E-02 *Ei/E0 = 7.9289447E-03
Iteration: 2 *|du|/|u| = 8.2394603E-03 *Ei/E0 = 3.7641070E-03
Iteration: 3 *|du|/|u| = 7.2058098E-04 *Ei/E0 = 1.2425847E-04
Equilibrium established after 3 iterations 06/26/25 18:15:05
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.35936E-05 dt(new) = 2.15443E-05
------------------------------------------------------------
BEGIN implicit dynamics step 80 t= 3.3169E-01 06/26/25 18:15:05
============================================================
time = 3.31686E-01
current step size = 2.15443E-05
Iteration: 1 *|du|/|u| = 2.5248570E-02 *Ei/E0 = 7.7772667E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 7.360E+03 exceeds prior maximum 3.264E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 1995 vs 2033
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 2.15443E-05 dt(new) = 1.00000E-05
------------------------------------------------------------
BEGIN implicit dynamics step 80 t= 3.3167E-01 06/26/25 18:15:05
============================================================
time = 3.31674E-01
current step size = 1.00000E-05
Iteration: 1 *|du|/|u| = 1.2206015E-02 *Ei/E0 = 7.9944852E-03
Iteration: 2 *|du|/|u| = 1.1201444E-02 *Ei/E0 = 7.3576689E-03
Iteration: 3 *|du|/|u| = 3.4749801E-04 *Ei/E0 = 4.1450566E-05
Equilibrium established after 3 iterations 06/26/25 18:15:06
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.00000E-05 dt(new) = 1.58489E-05
------------------------------------------------------------
BEGIN implicit dynamics step 81 t= 3.3169E-01 06/26/25 18:15:06
============================================================
time = 3.31690E-01
current step size = 1.58489E-05
Iteration: 1 *|du|/|u| = 1.8734026E-02 *Ei/E0 = 7.8909530E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 6.171E+03 exceeds prior maximum 3.758E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 8.5827992E-04 *Ei/E0 = 4.3177248E-04
Equilibrium established after 2 iterations 06/26/25 18:15:06
------------------------------------------------------------
Converged in less than ITEOPT-ITEWIN= 6 iterations,
automatic time step size INCREASE:
dt(old) = 1.58489E-05 dt(new) = 2.51189E-05
------------------------------------------------------------
BEGIN implicit dynamics step 82 t= 3.3171E-01 06/26/25 18:15:06
============================================================
time = 3.31715E-01
current step size = 2.51189E-05
Iteration: 1 *|du|/|u| = 2.7977438E-02 *Ei/E0 = 7.2296242E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 3.764E+03 exceeds prior maximum 2.393E+03
automatically REFORMING stiffness matrix...
Iteration: 2 *|du|/|u| = 2.0573369E-02 *Ei/E0 = 4.2113952E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 1.778E+04 exceeds prior maximum 3.764E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 44 vs 46
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 2.51189E-05 dt(new) = 1.16591E-05
------------------------------------------------------------
BEGIN implicit dynamics step 82 t= 3.3170E-01 06/26/25 18:15:07
============================================================
time = 3.31701E-01
current step size = 1.16591E-05
Iteration: 1 *|du|/|u| = 1.3466995E-02 *Ei/E0 = 7.5927785E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 2.011E+04 exceeds prior maximum 4.881E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 44 vs 46
------------------------------------------------------------
automatic time step size DECREASE, RETRY step:
dt(old) = 1.16591E-05 dt(new) = 1.00000E-05
------------------------------------------------------------
BEGIN implicit dynamics step 82 t= 3.3170E-01 06/26/25 18:15:07
============================================================
time = 3.31700E-01
current step size = 1.00000E-05
Iteration: 1 *|du|/|u| = 1.1625407E-02 *Ei/E0 = 7.6337813E-03
DIVERGENCE (increasing translational residual norm) detected:
current norm 9.904E+03 exceeds prior maximum 5.662E+03
automatically REFORMING stiffness matrix...
Contact algorithm rejected current iterate,
list of element pairs affected follows (at most 10):
Element # 44 vs 46
*** Error 60004 (IMP+4)
*********************************************************
* *
* - FATAL ERROR - *
* *
* Nonlinear solver failed to find equilibrium. *
* *
*********************************************************
Writing out last converged state for implicit.
4315 t 3.3169E-01 dt 0.00E+00 write d3plot file 06/26/25 18:15:08
E r r o r t e r m i n a t i o n 06/26/25 18:15:08
Memory required to complete solution (memory= 354K)
Minimum 311K on processor 1
Maximum 354K on processor 0
Average 332K
Matrix Assembly dynamically allocated memory
Maximum 873K
Additional dynamically allocated memory
Minimum 15M on processor 1
Maximum 17M on processor 0
Average 16M
Total allocated memory
Minimum 17M on processor 1
Maximum 18M on processor 0
Average 17M
T i m i n g i n f o r m a t i o n
CPU(seconds) %CPU Clock(seconds) %Clock
----------------------------------------------------------------
Keyword Processing ... 2.1994E-02 0.02 2.2022E-02 0.01
KW Reading ......... 5.3565E-03 0.00 5.3565E-03 0.00
MPP Decomposition .... 2.9838E-02 0.02 3.0898E-02 0.02
Init Proc .......... 1.8509E-02 0.01 1.9188E-02 0.01
Decomposition ...... 2.2539E-03 0.00 2.2535E-03 0.00
Translation ........ 9.0296E-03 0.01 9.4090E-03 0.01
Initialization ....... 3.6875E+00 2.56 7.2637E+00 4.91
Init Proc Phase 1 .. 1.4672E-02 0.01 1.5089E-02 0.01
Init Proc Phase 2 .. 2.4498E-02 0.02 2.5375E-02 0.02
Element processing ... 2.8958E+01 20.14 2.8959E+01 19.57
Shells ............. 2.8207E+01 19.62 2.8207E+01 19.06
E Other ............ 2.6447E-02 0.02 2.7361E-02 0.02
Binary databases ..... 3.2531E-01 0.23 3.2532E-01 0.22
ASCII database ....... 1.5716E-02 0.01 1.5785E-02 0.01
Contact algorithm .... 5.5392E+01 38.52 5.5396E+01 37.44
Interf. ID 1 5.5322E+01 38.47 5.5322E+01 37.39
Rigid Bodies ......... 3.4643E-02 0.02 3.4449E-02 0.02
Implicit ............. 2.9385E-01 0.20 2.9610E-01 0.20
Implicit Nonlinear ... 1.9562E+01 13.60 2.0095E+01 13.58
Imp. NL force mod .. 1.6707E+01 11.62 1.6705E+01 11.29
Implicit Lin. Alg. ... 3.3710E+01 23.44 3.3778E+01 22.83
Imp. LA Mtx Assemb . 1.5816E+01 11.00 1.5816E+01 10.69
Imp. LA Factor ..... 1.5357E+01 10.68 1.5358E+01 10.38
Imp. LA Solve ...... 2.5028E+00 1.74 2.5690E+00 1.74
Time step size ....... 1.4941E+00 1.04 1.4941E+00 1.01
Others ............... 1.0664E-01 0.07 1.0690E-01 0.07
Force Sharing ...... 6.4176E-02 0.04 6.3924E-02 0.04
Misc. 1 .............. 7.2355E-02 0.05 7.3635E-02 0.05
Scale Masses ....... 4.1174E-02 0.03 4.0982E-02 0.03
Force Constraints .. 5.5093E-03 0.00 5.7700E-03 0.00
Misc. 3 .............. 2.1201E-03 0.00 2.1105E-03 0.00
Misc. 4 .............. 8.3954E-02 0.06 8.4158E-02 0.06
Timestep Init ...... 3.3348E-02 0.02 3.2948E-02 0.02
Apply Loads ........ 3.1379E-02 0.02 3.1457E-02 0.02
Compute exwork ..... 3.9943E-03 0.00 4.0280E-03 0.00
----------------------------------------------------------------
T o t a l s 1.4379E+02 100.00 1.4798E+02 100.00
Problem time = 3.3169E-01
Problem cycle = 4315
Total CPU time = 144 seconds ( 0 hours 2 minutes 24 seconds)
CPU time per zone cycle = 10025.715 nanoseconds
Clock time per zone cycle= 9818.724 nanoseconds
Parallel execution with 2 MPP proc
NLQ used/max 72/ 72
C P U T i m i n g i n f o r m a t i o n
Processor Hostname CPU/Avg_CPU CPU(seconds)
---------------------------------------------------------------------------
# 0 ebf4d118d8e6 0.97462 1.4016E+02
# 1 ebf4d118d8e6 1.02538 1.4746E+02
---------------------------------------------------------------------------
T o t a l s 2.8762E+02
Start time 06/26/2025 18:12:47
End time 06/26/2025 18:15:08
Elapsed time 141 seconds for 4315 cycles using 2 MPP procs
( 0 hour 2 minutes 21 seconds)
E r r o r t e r m i n a t i o n 06/26/25 18:15:08
4315 t 3.3169E-01 dt 0.00E+00 flush i/o buffers 06/26/25 18:15:08
Total running time of the script: (2 minutes 34.637 seconds)